SLS Lecture 10 : Assembly : Program Anatomy I
Contents
10. SLS Lecture 10 : Assembly : Program Anatomy I#
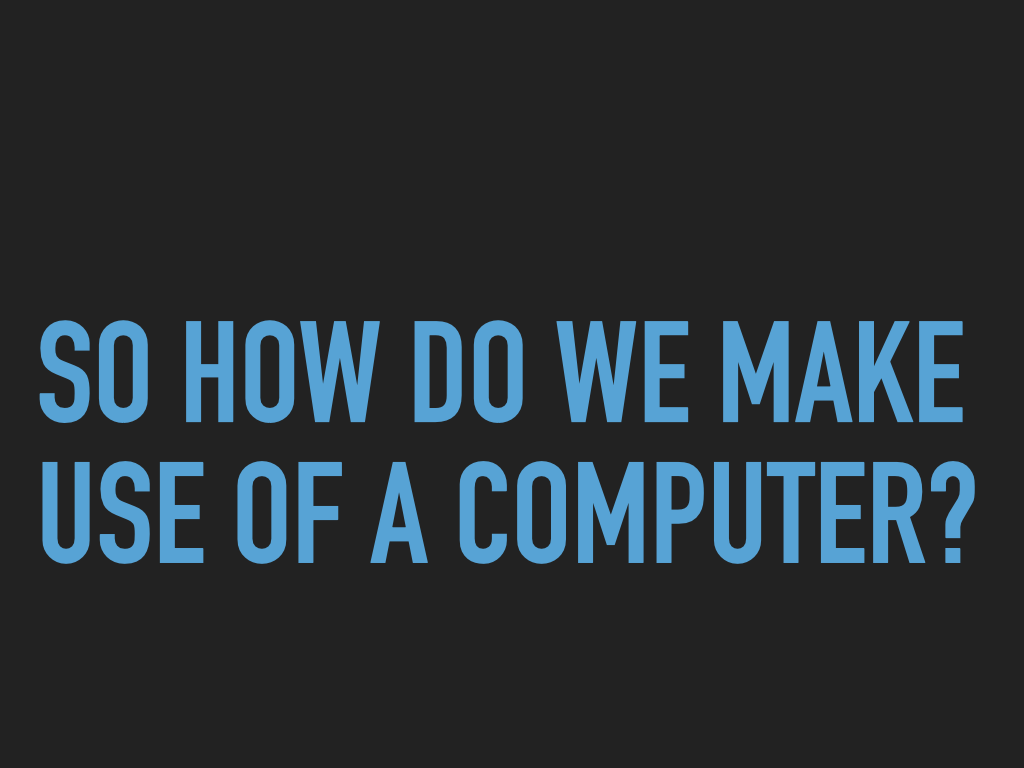
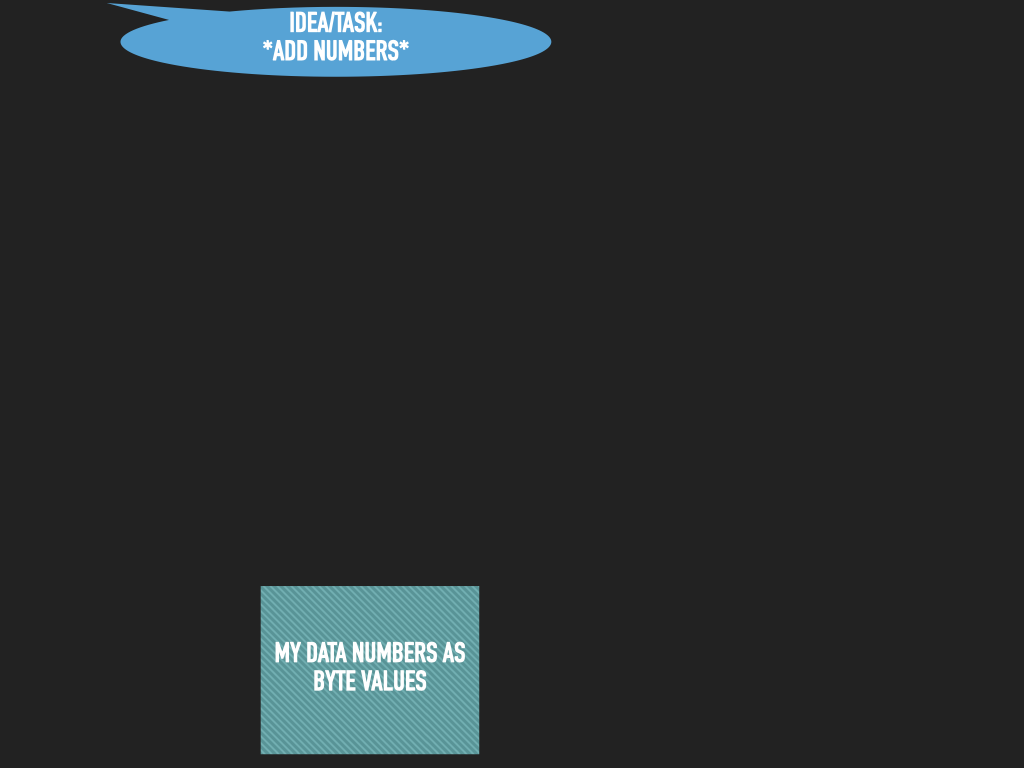
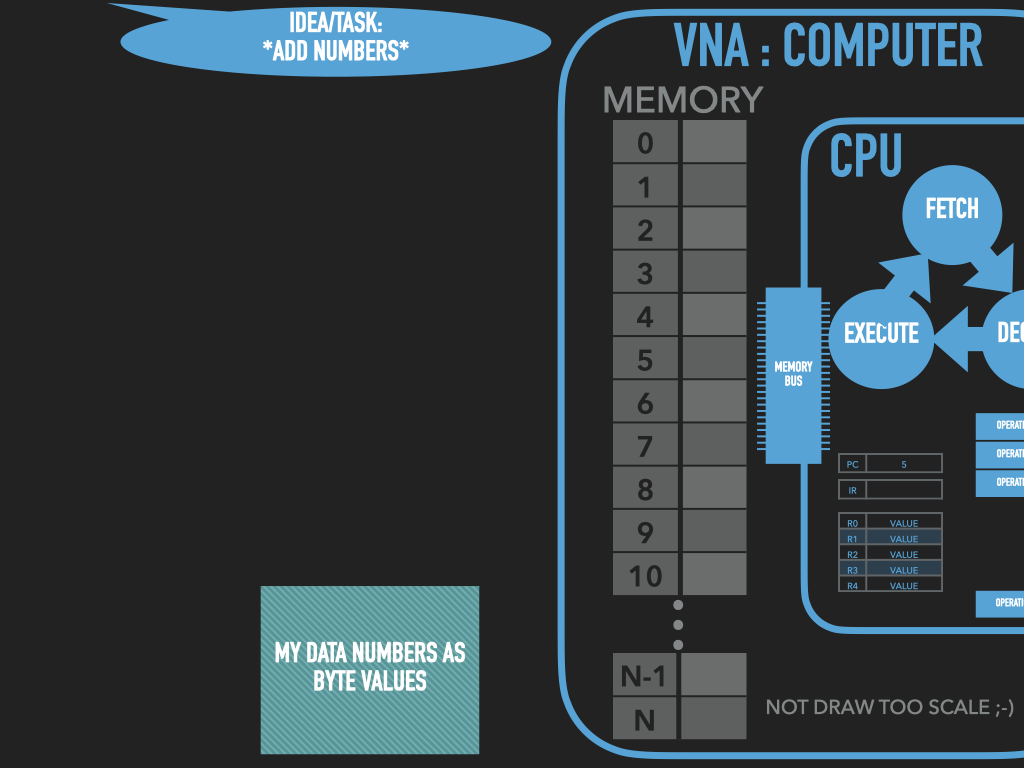
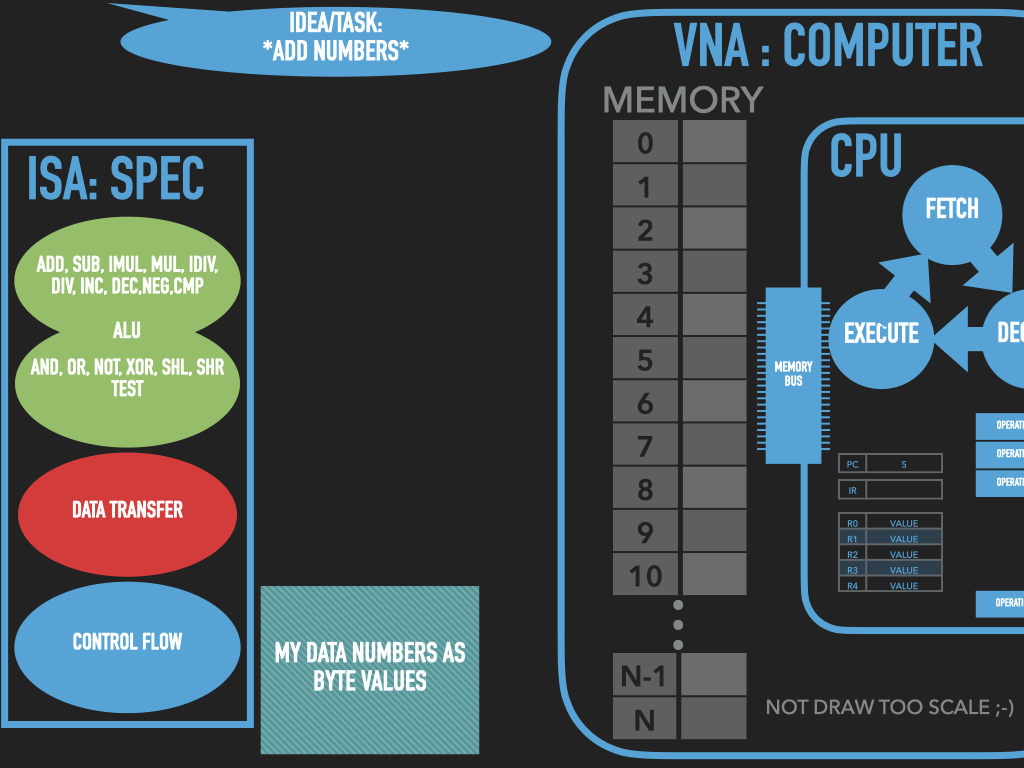
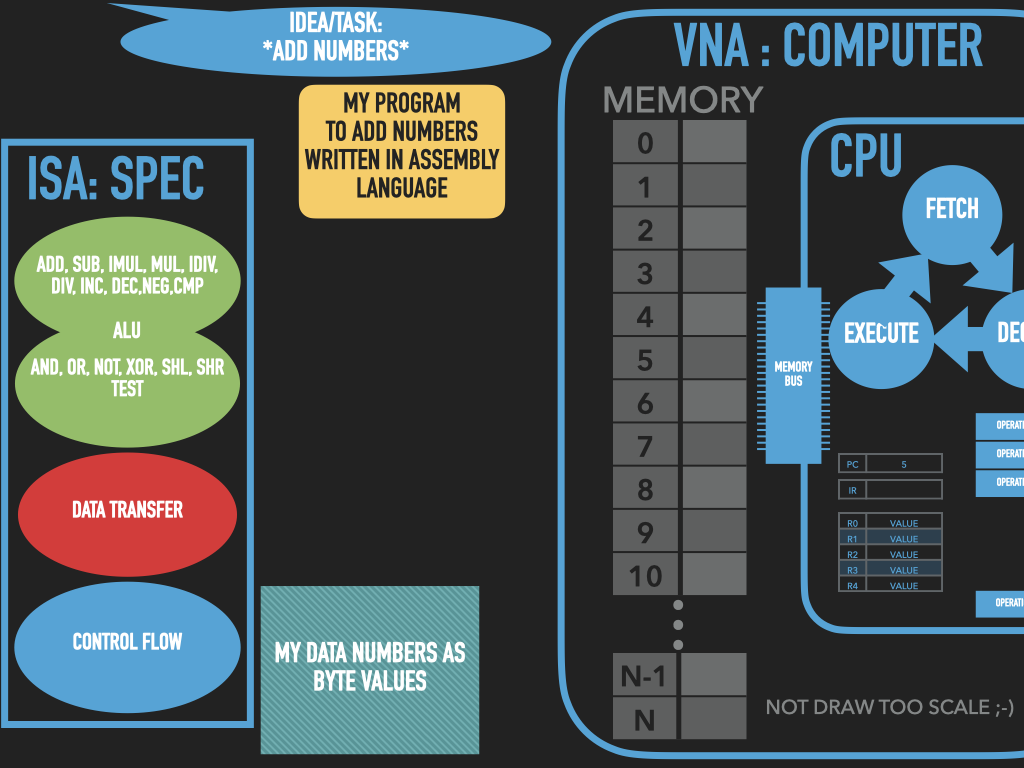
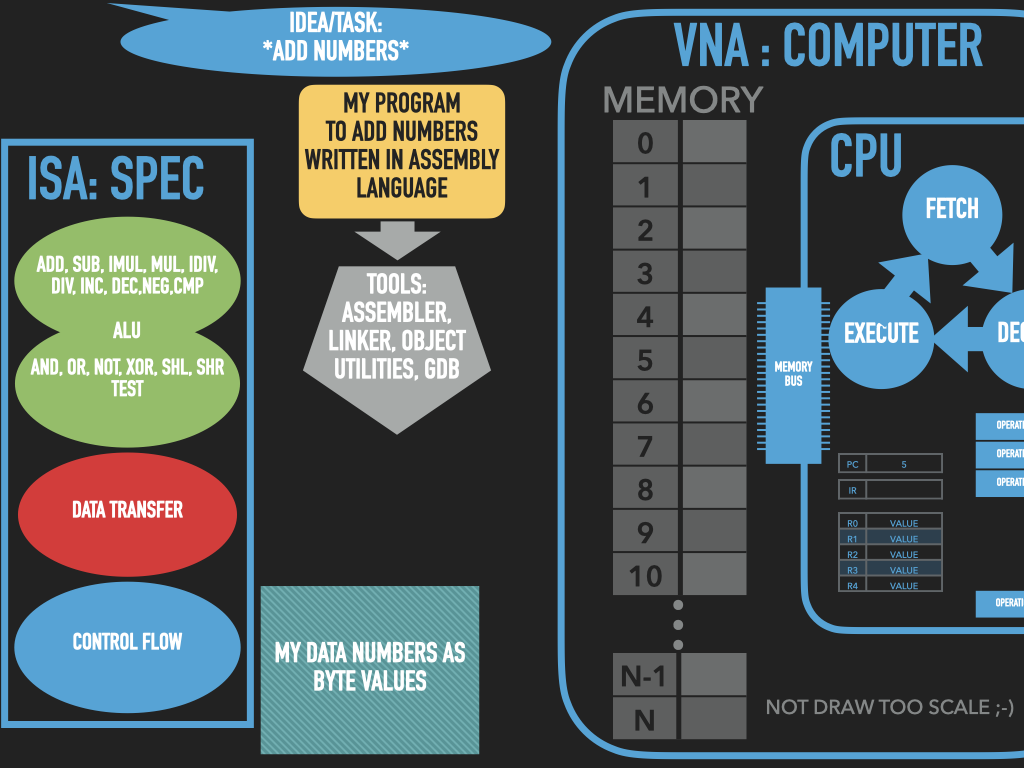
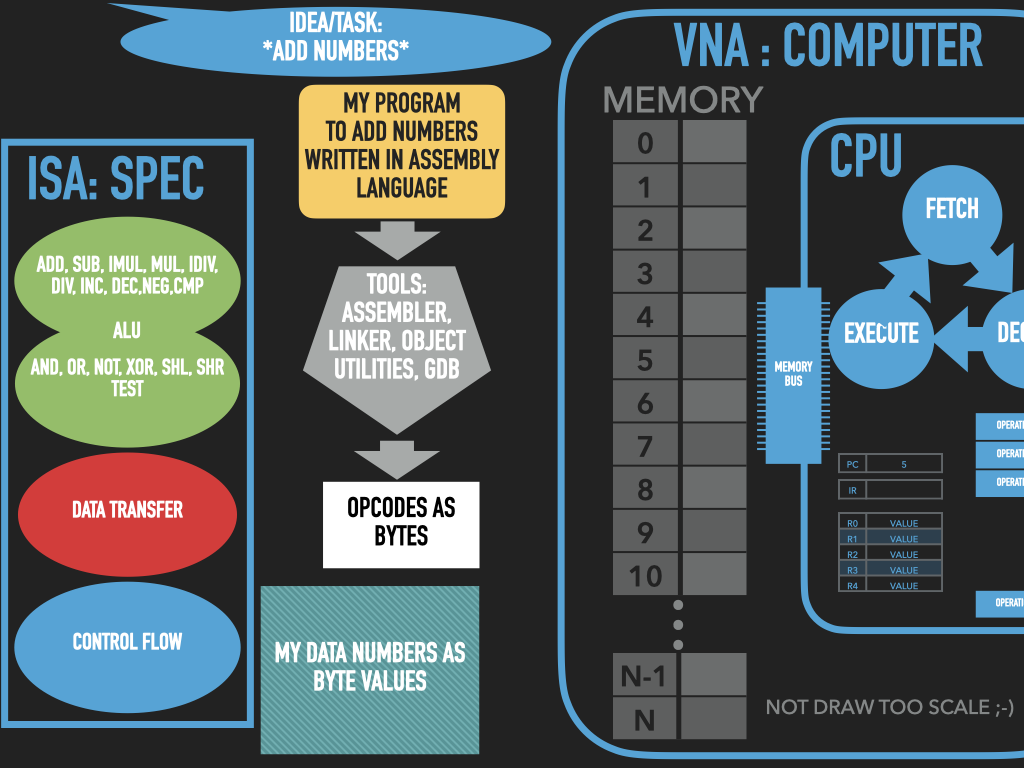
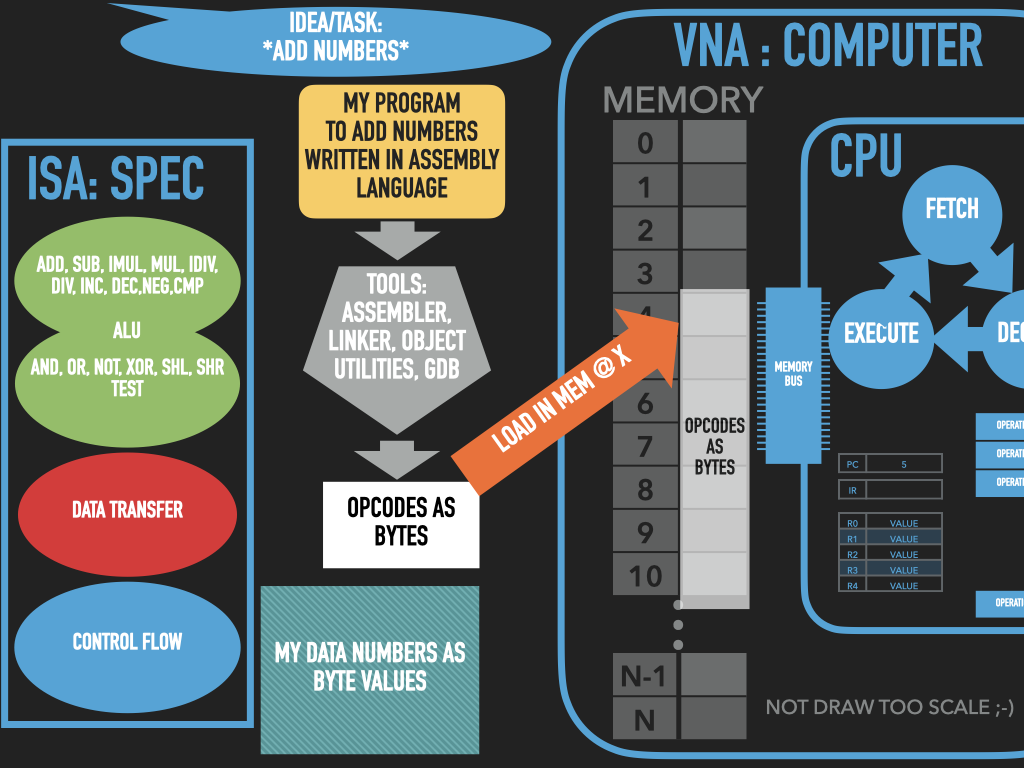
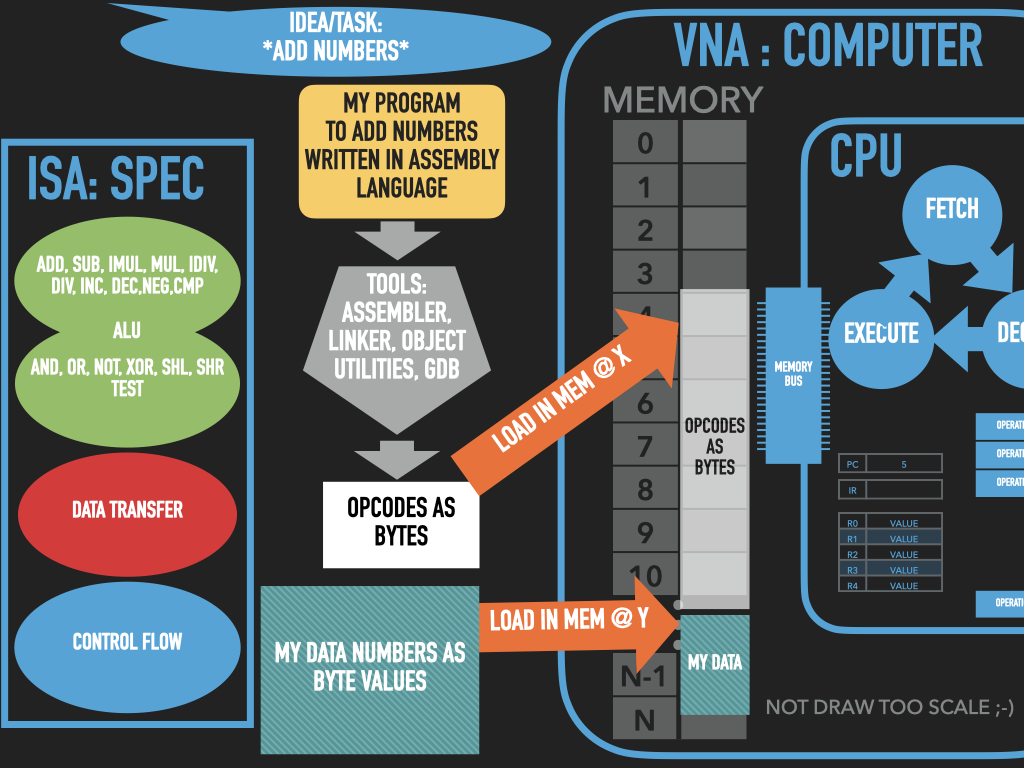
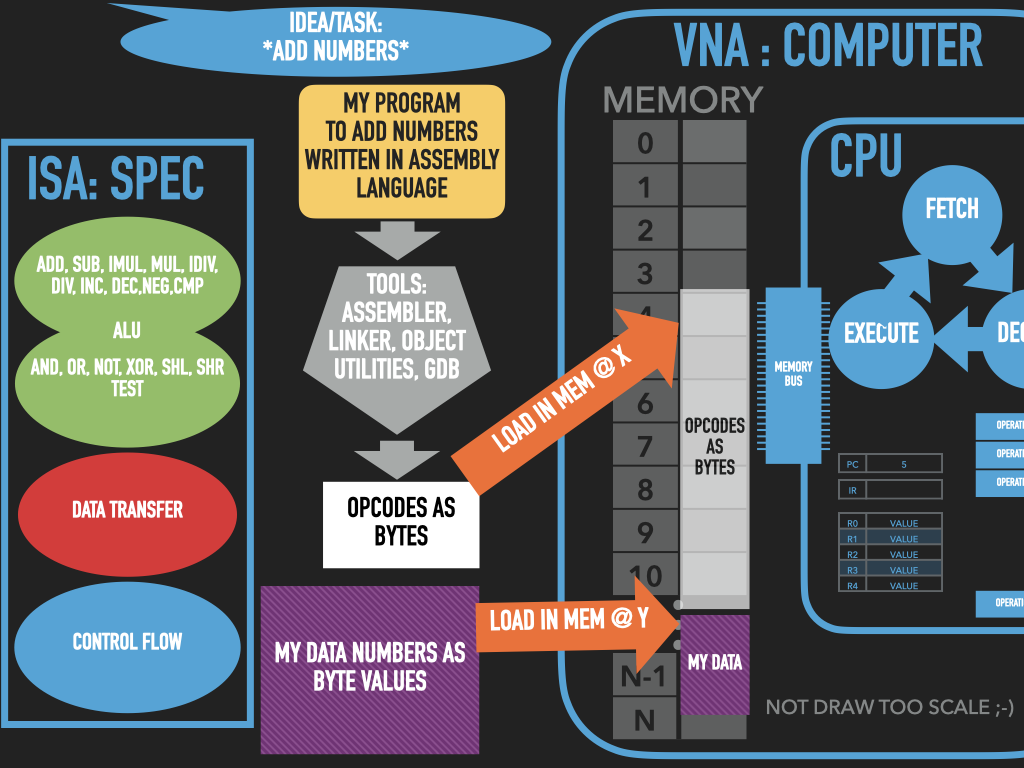
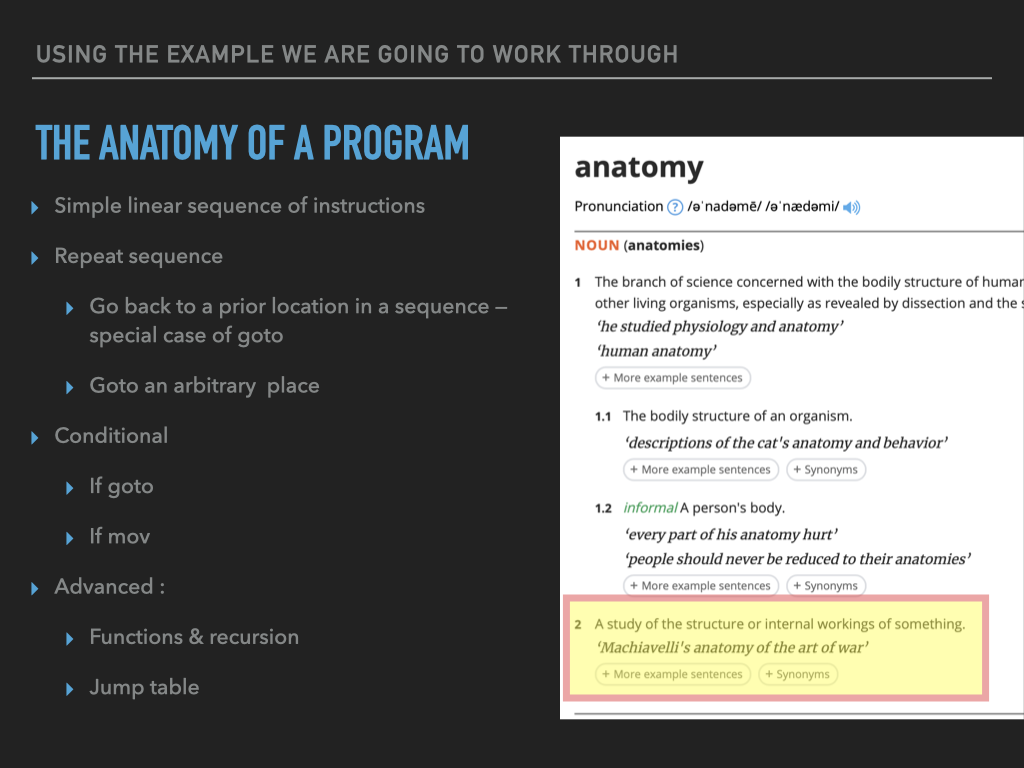
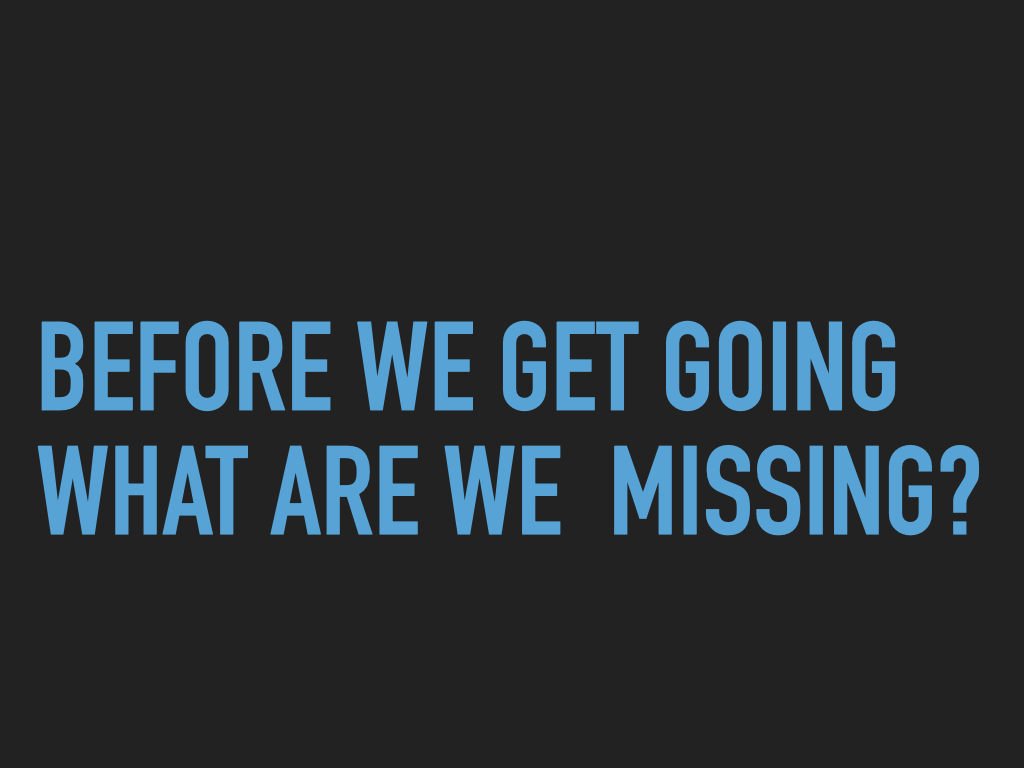
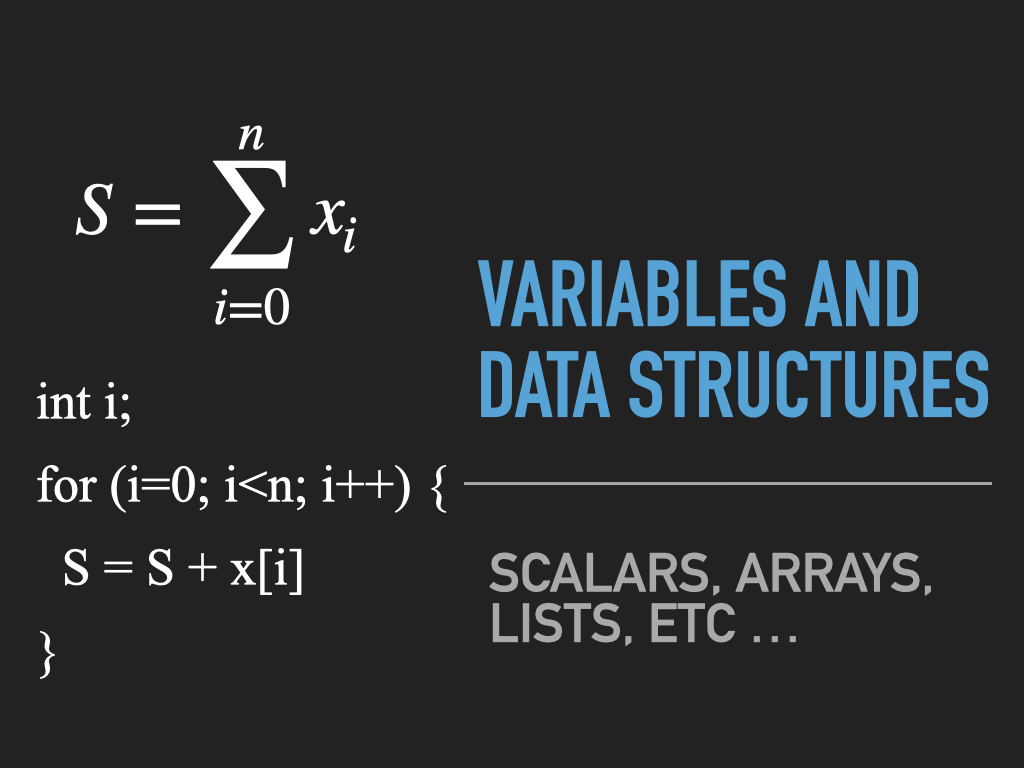
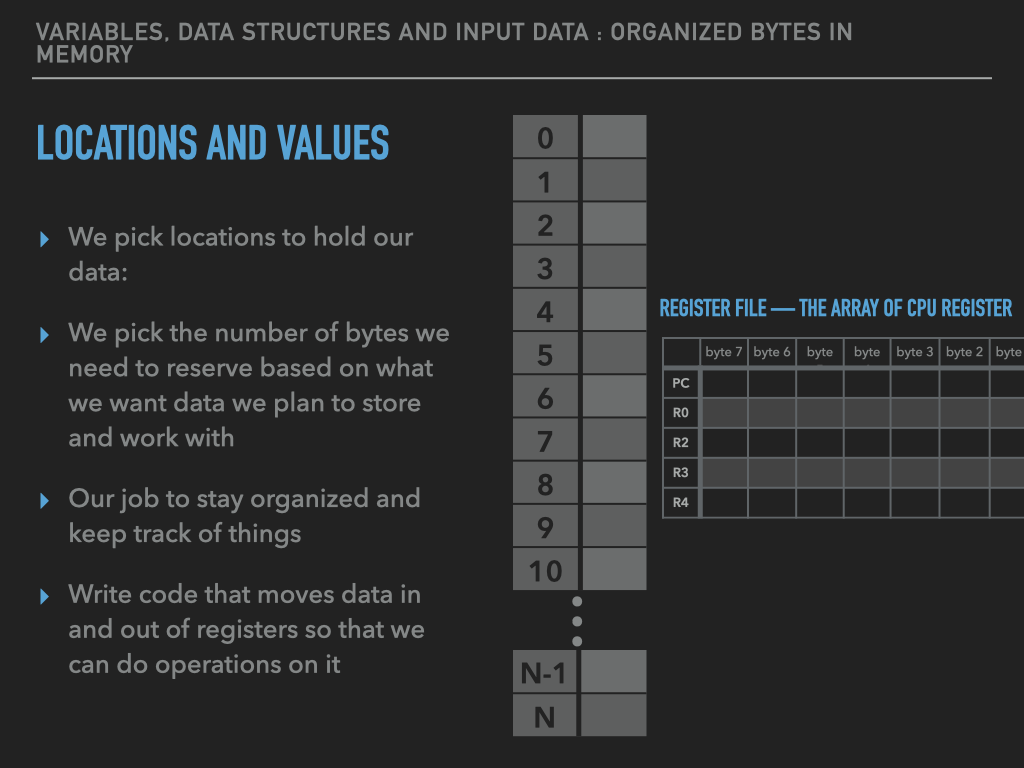
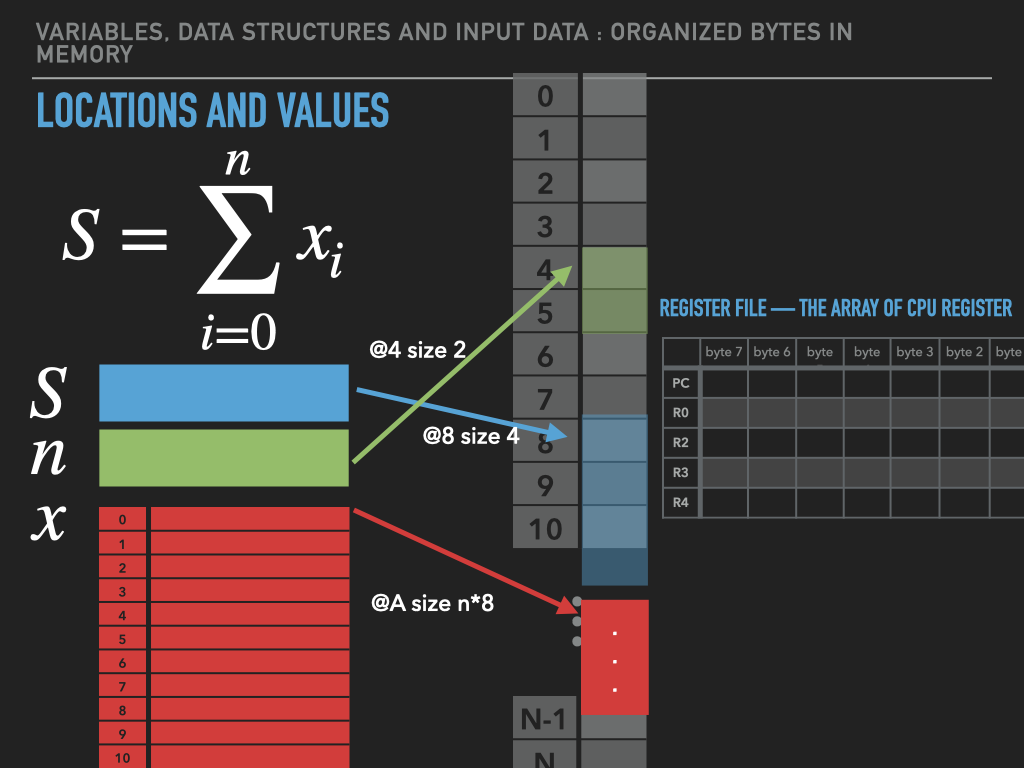
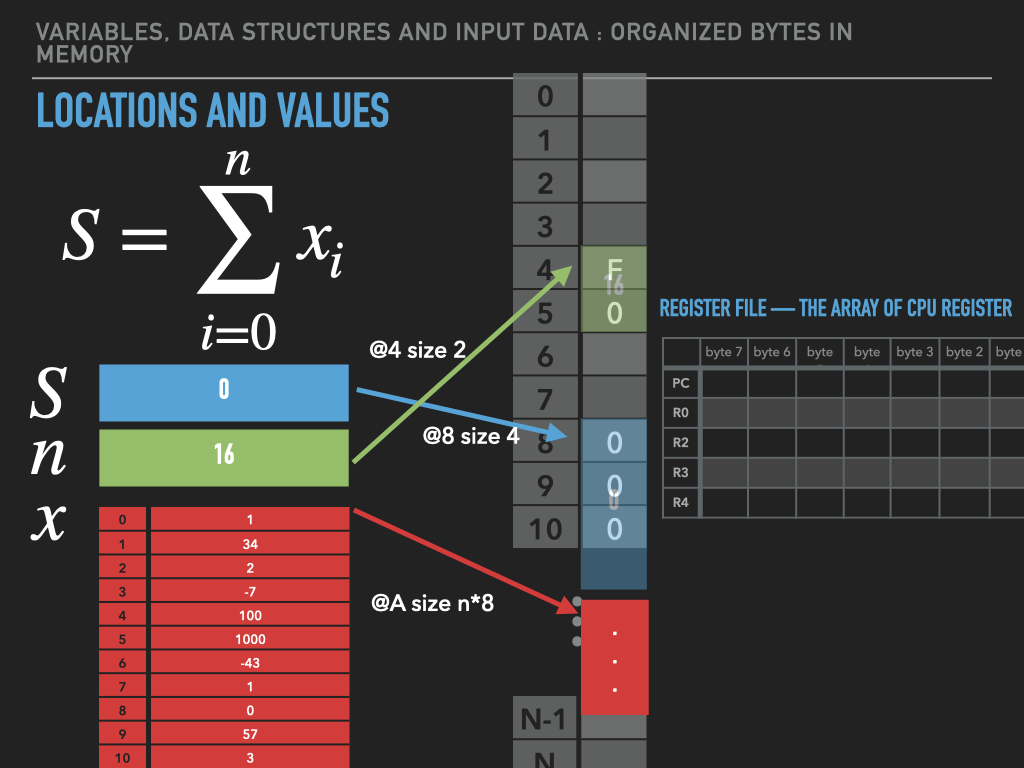
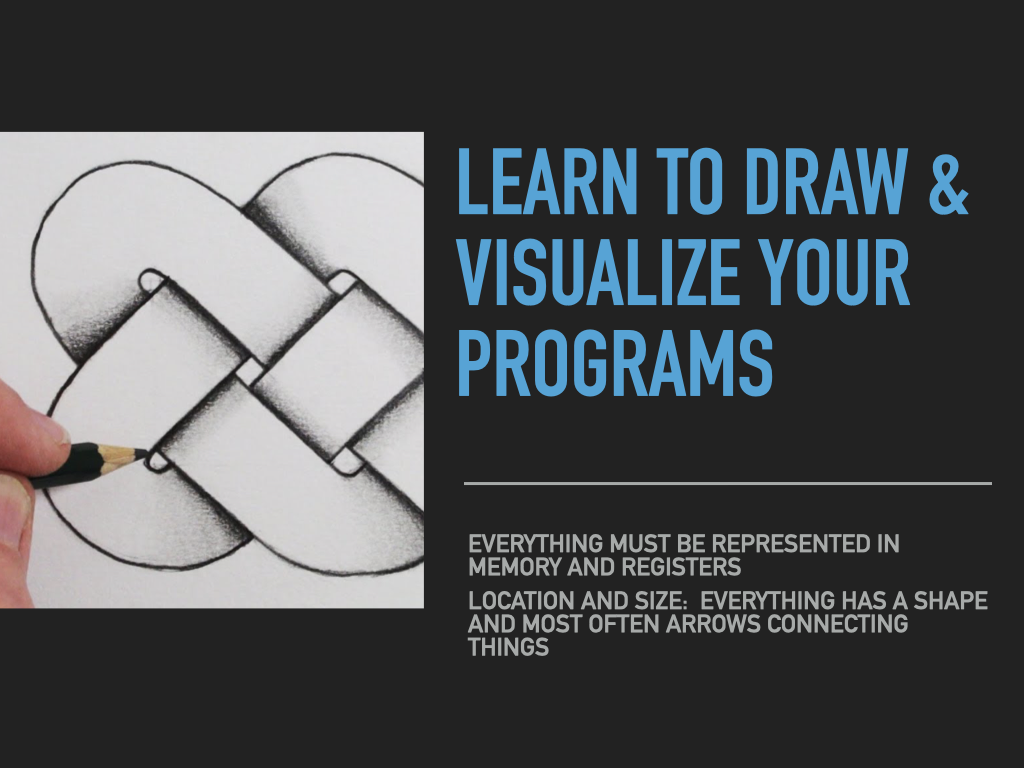
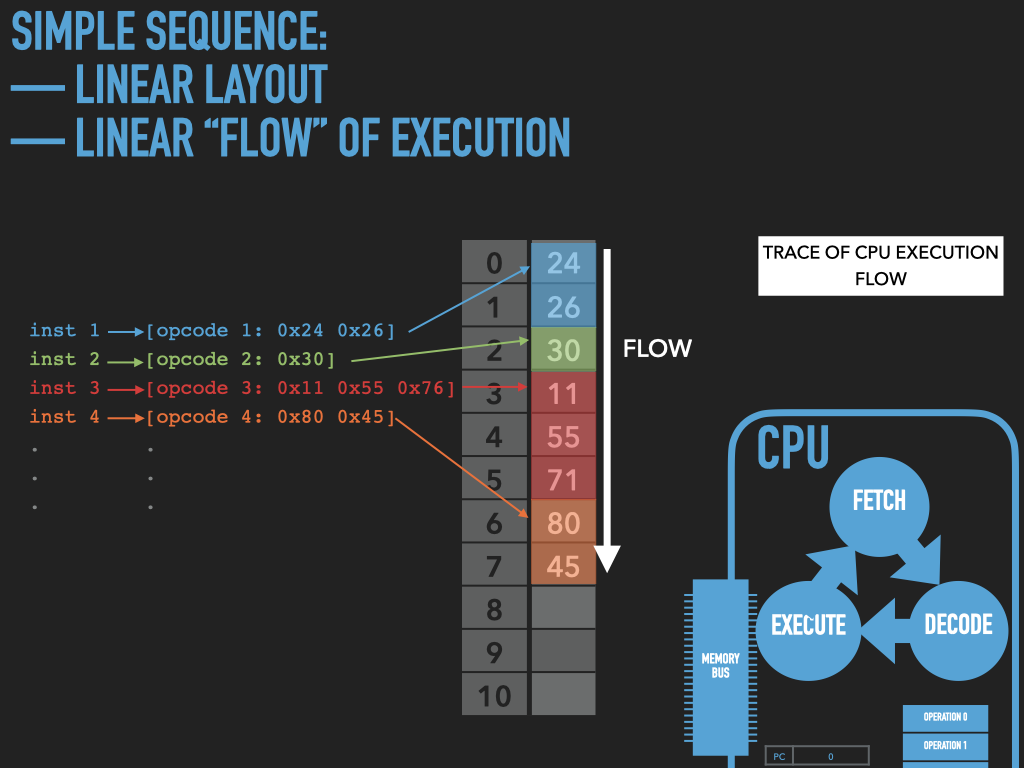
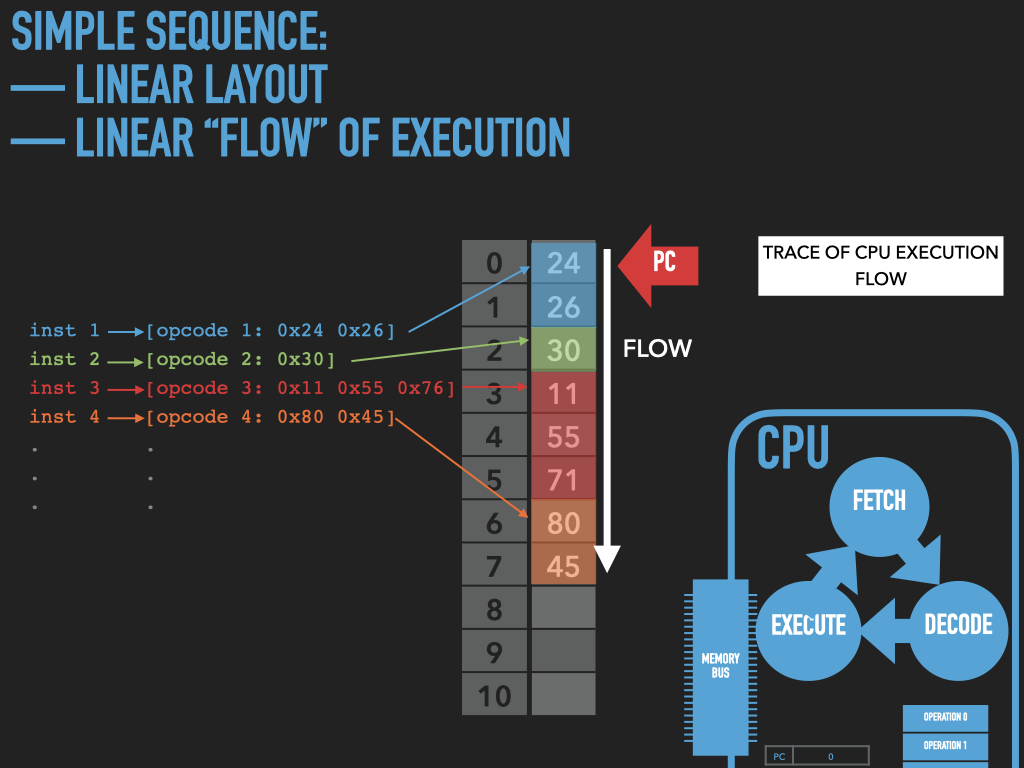
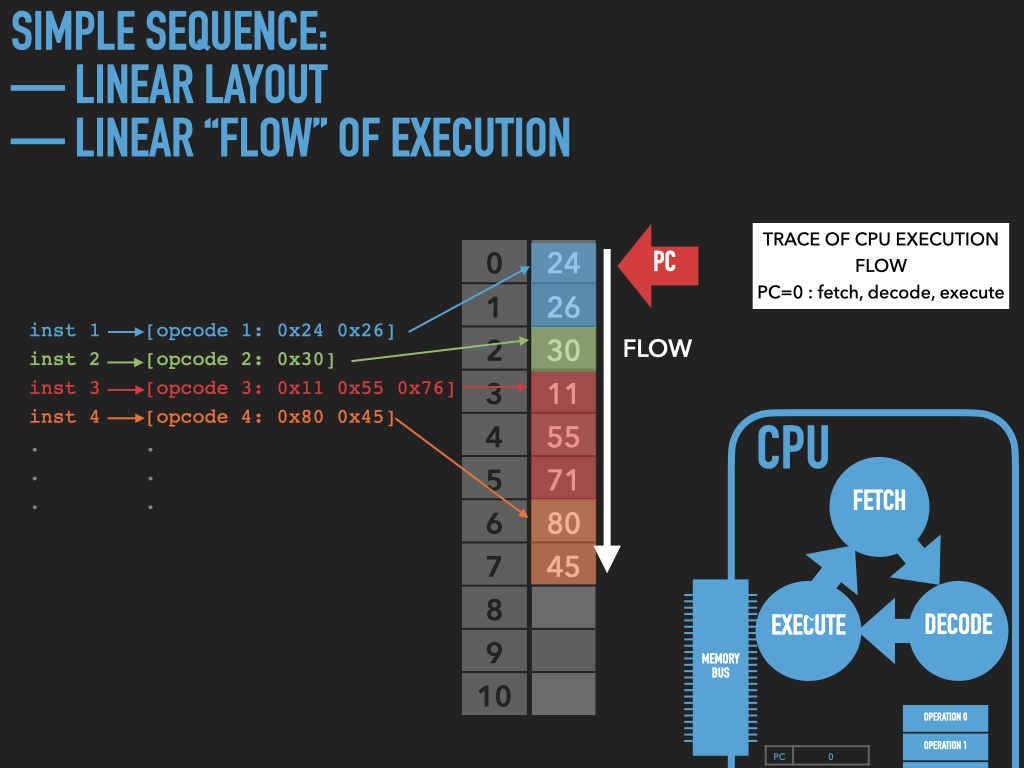
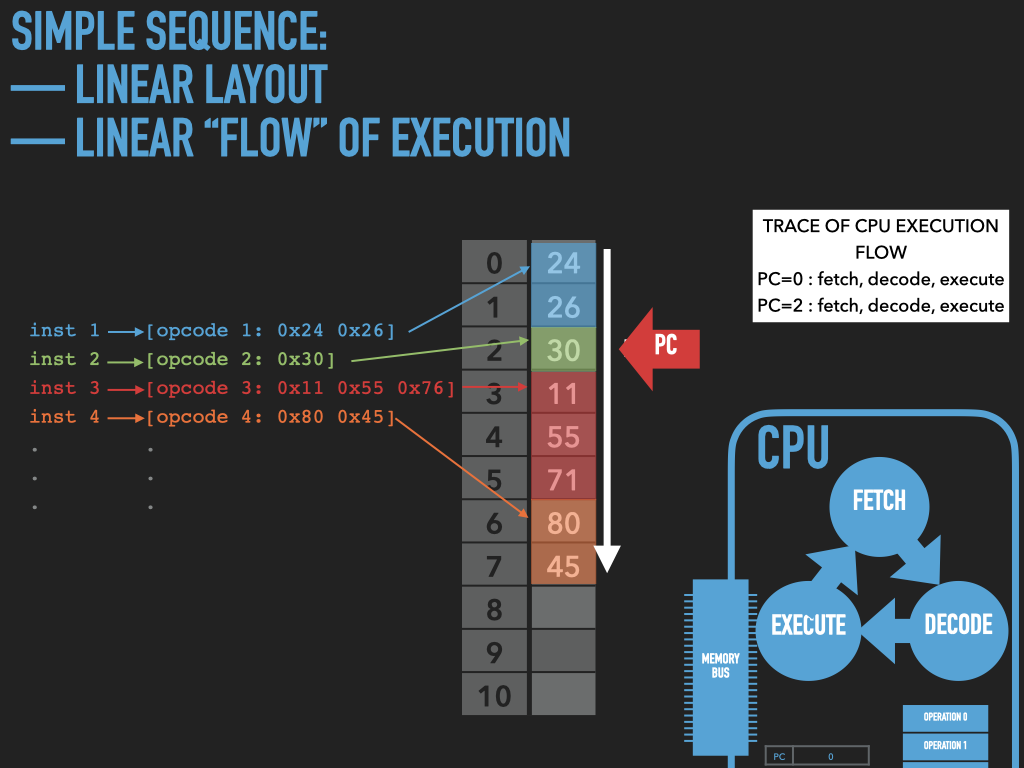
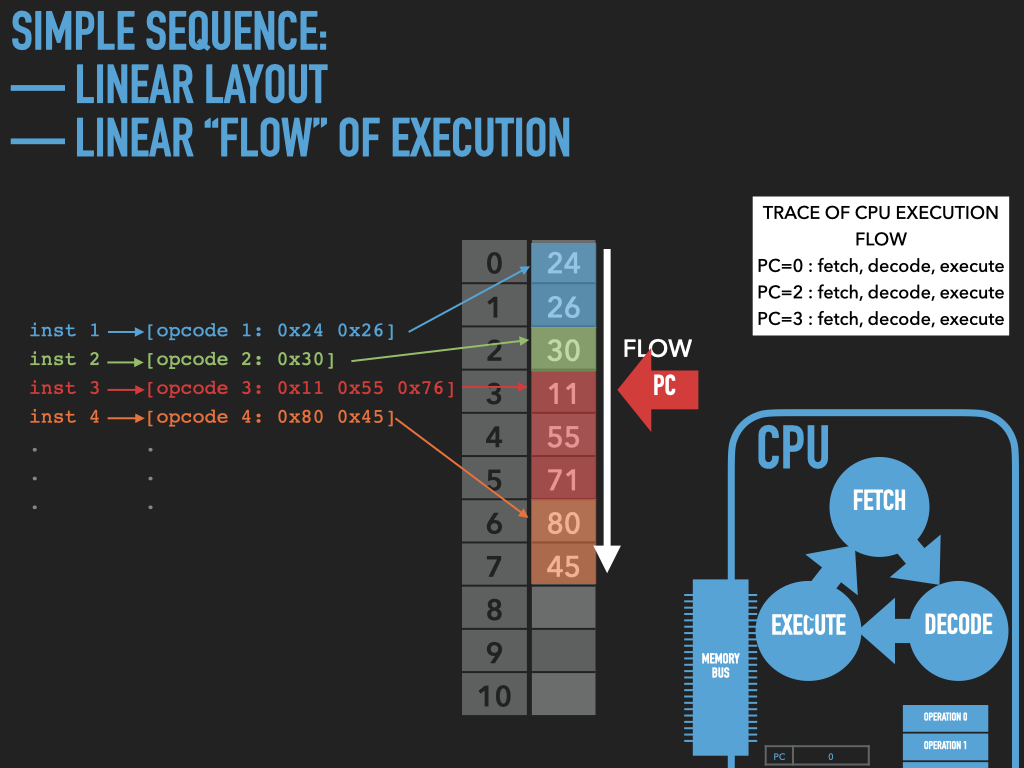
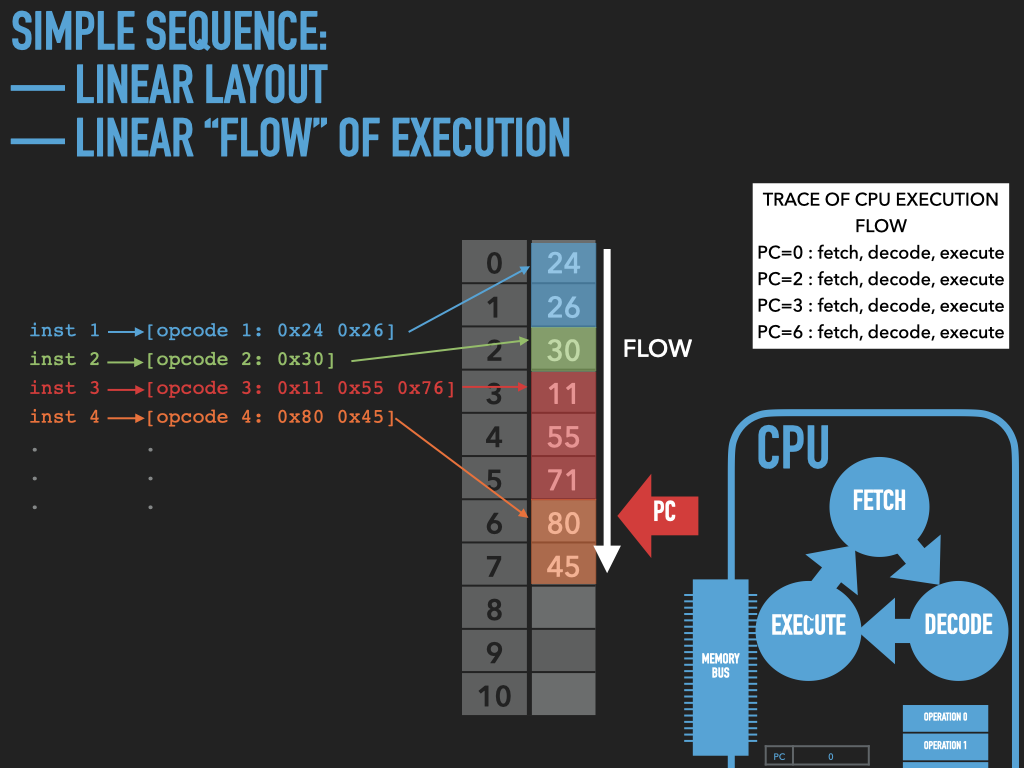
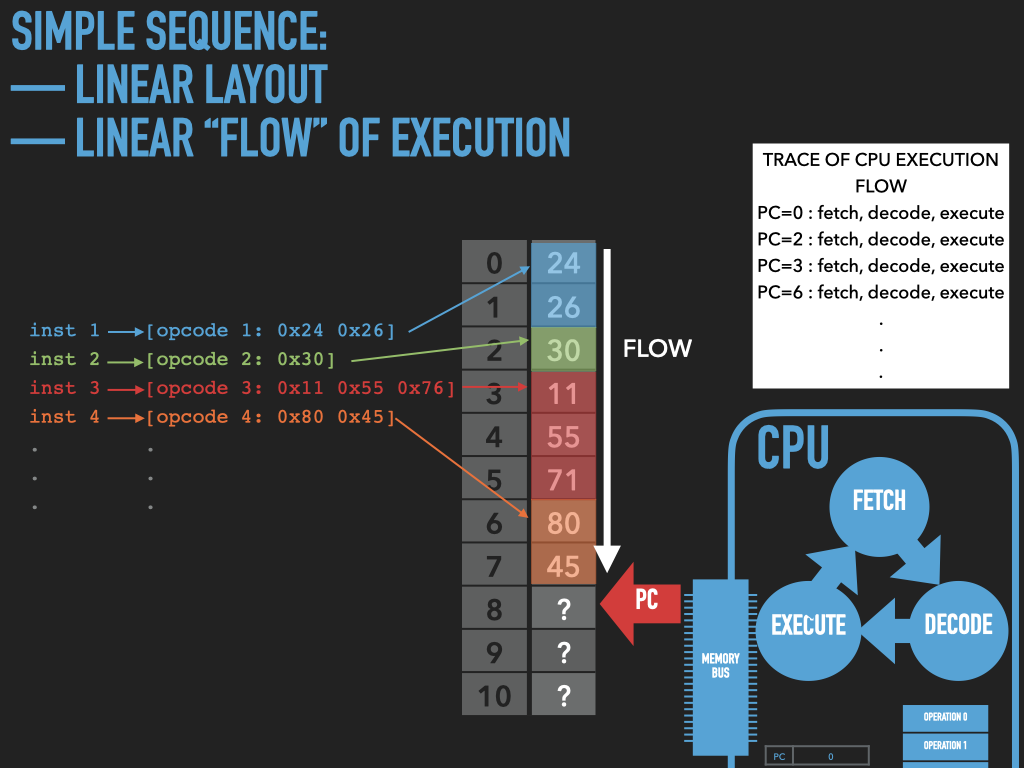
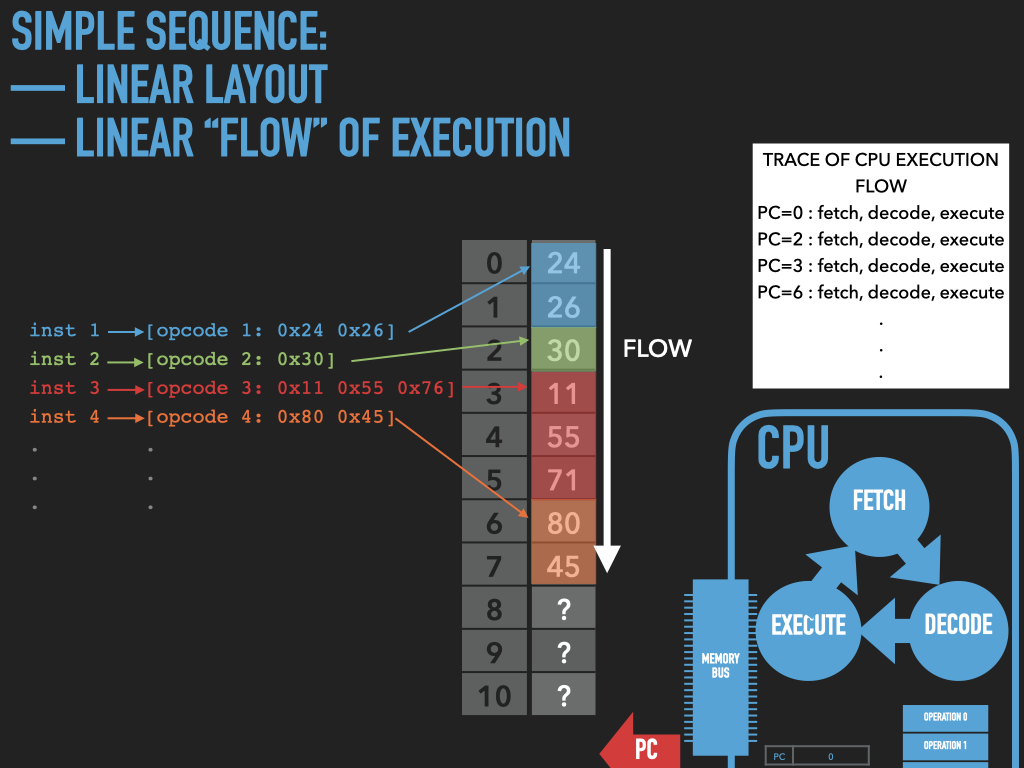
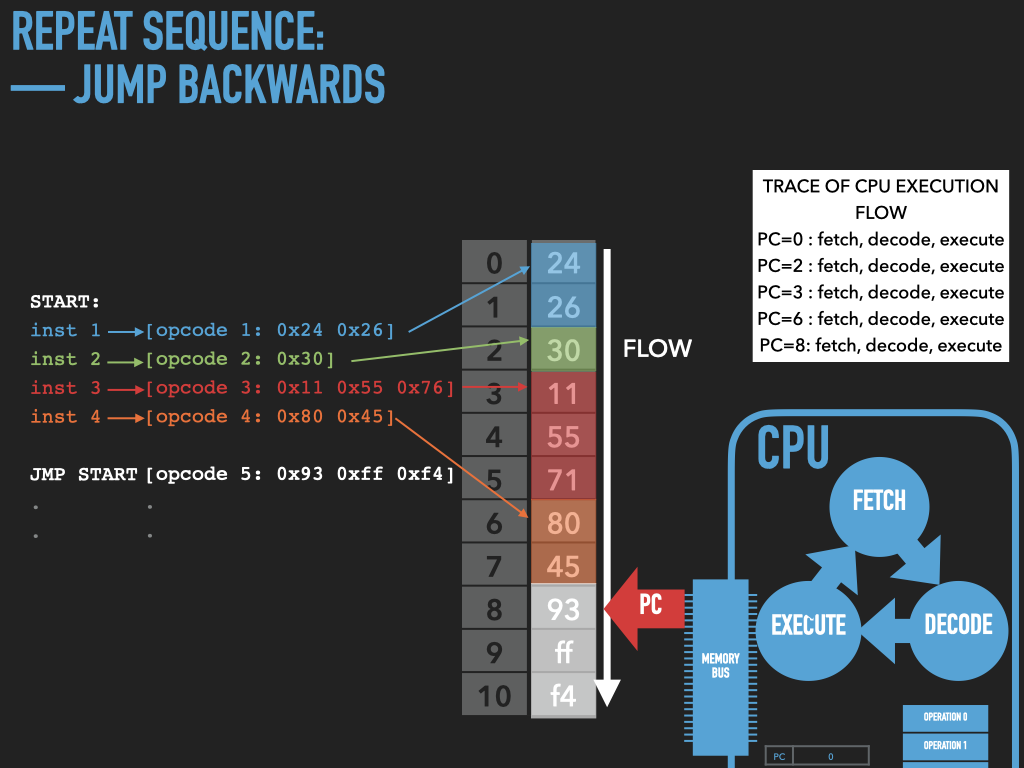
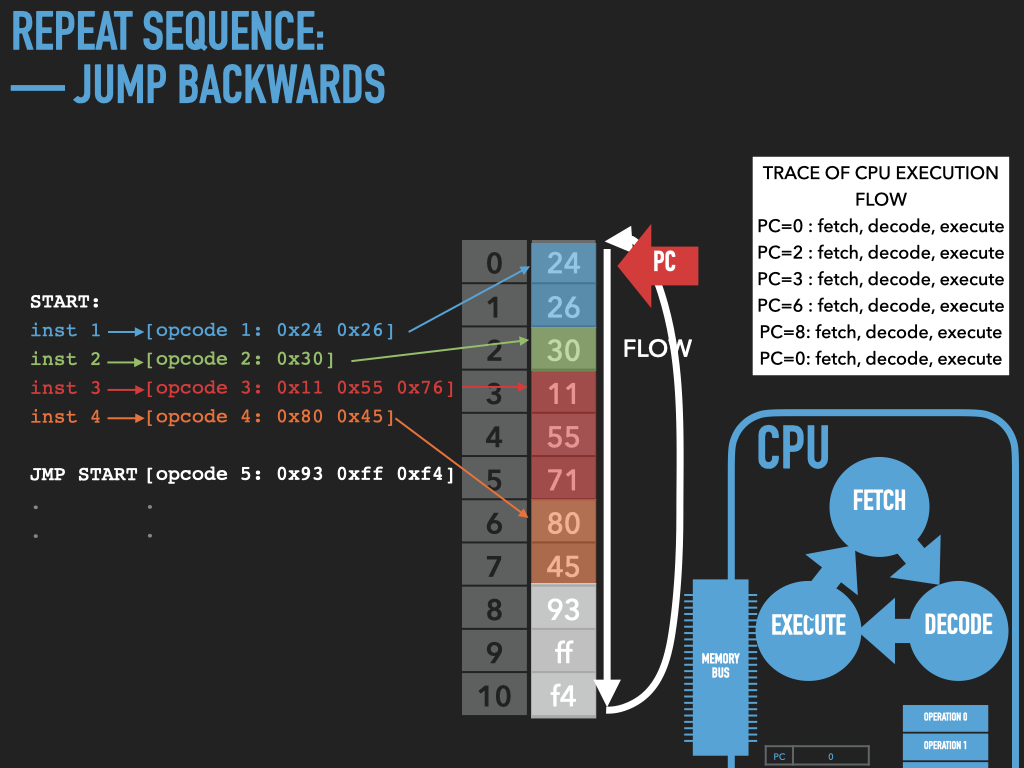
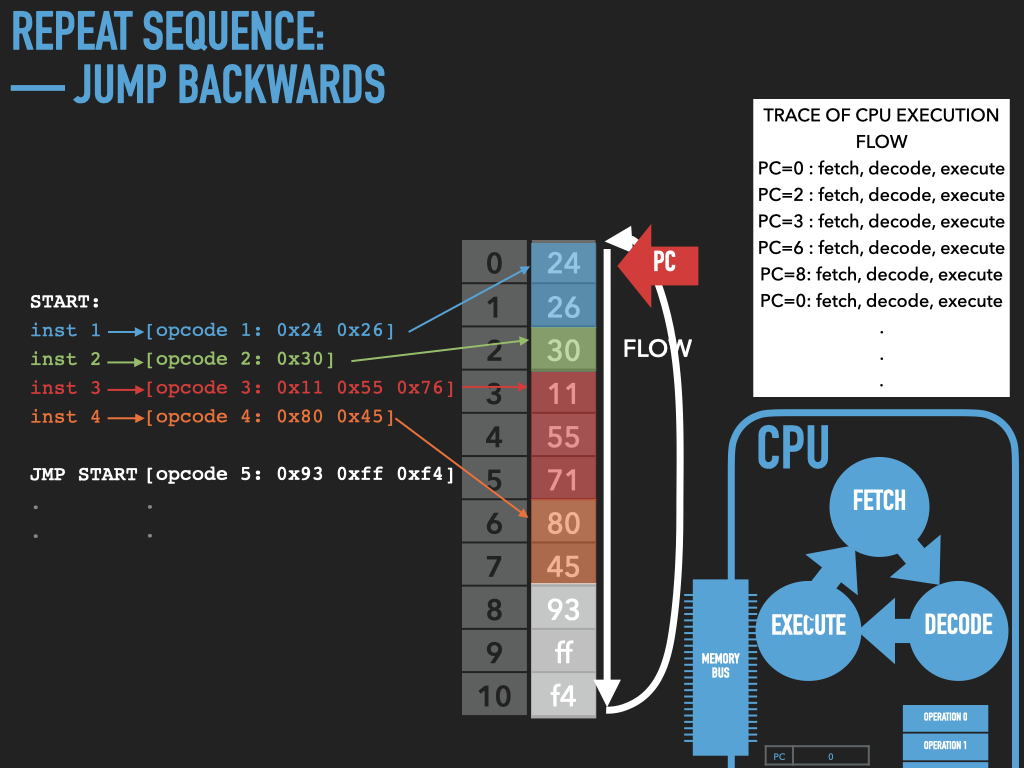
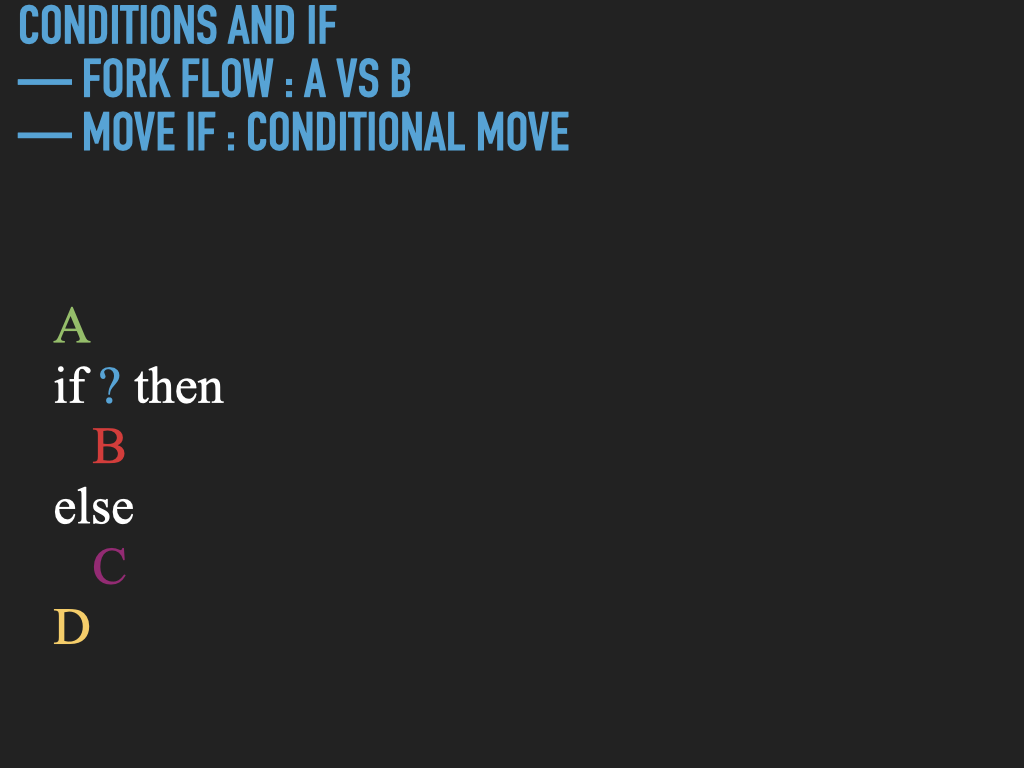
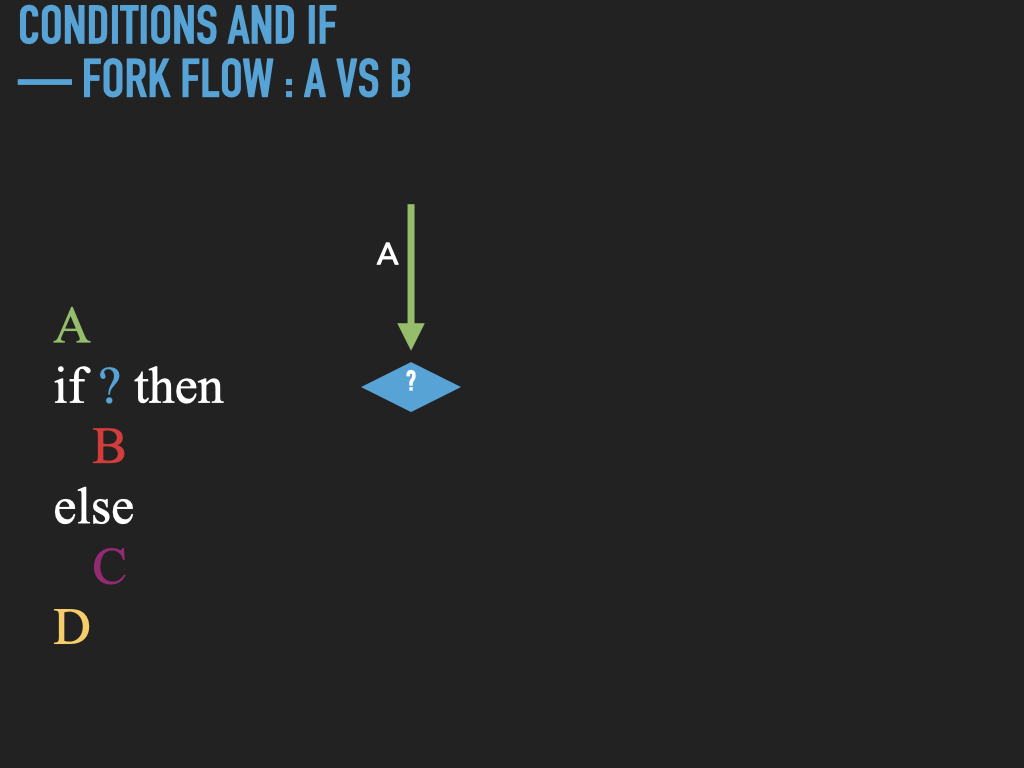
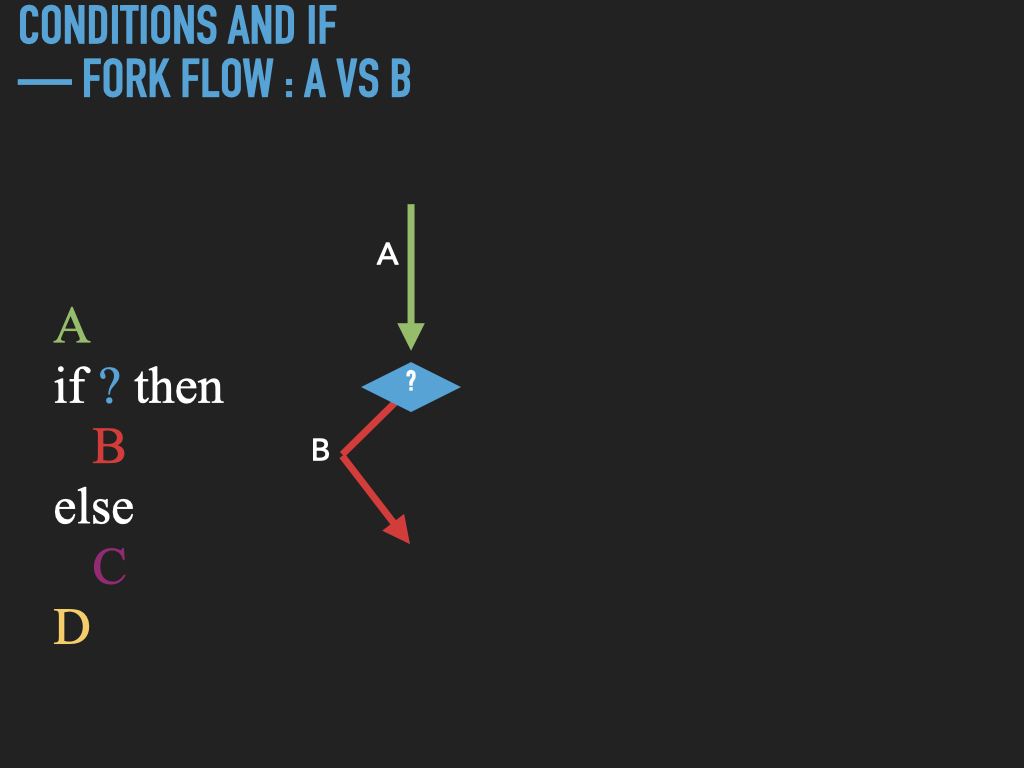
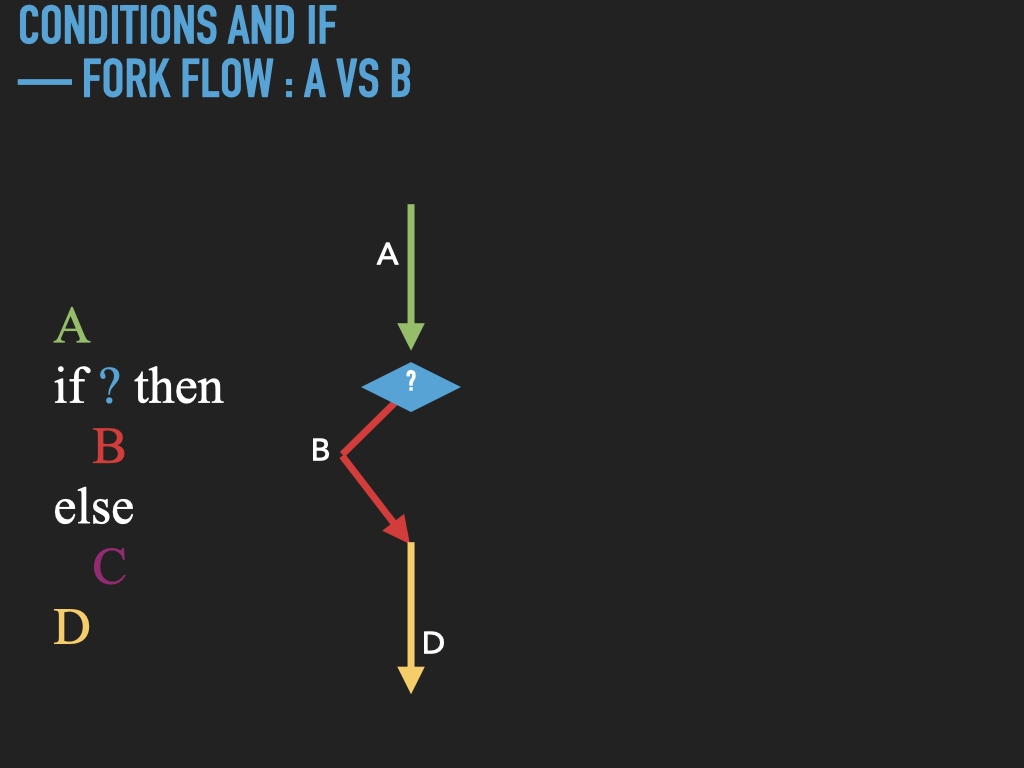
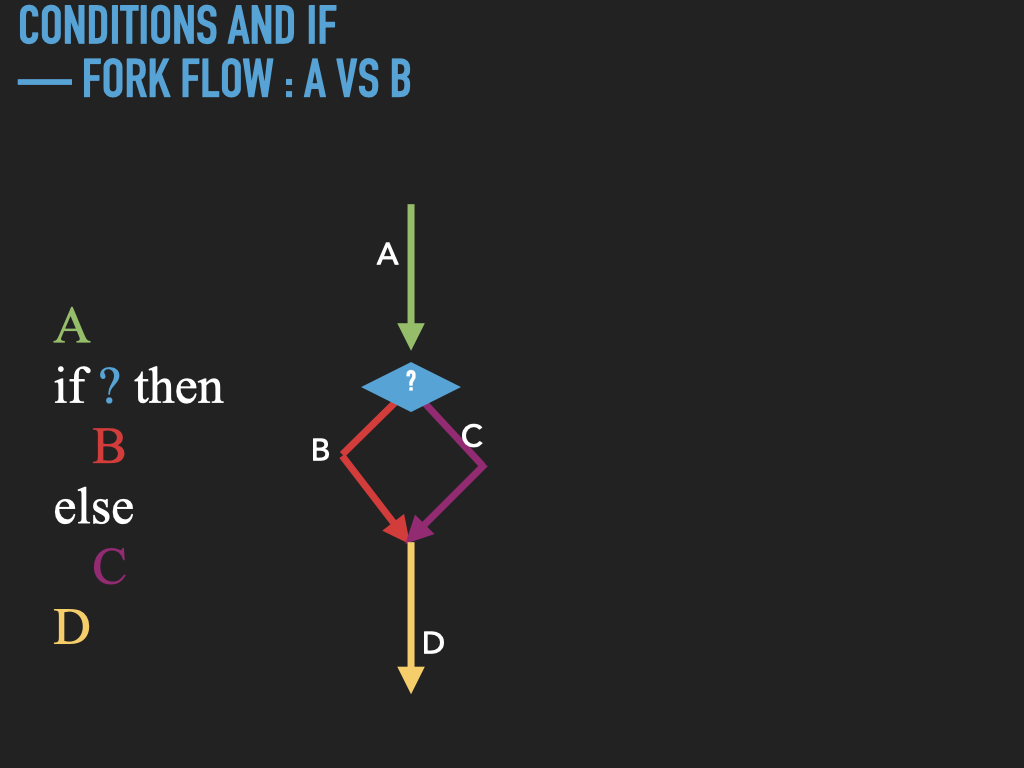
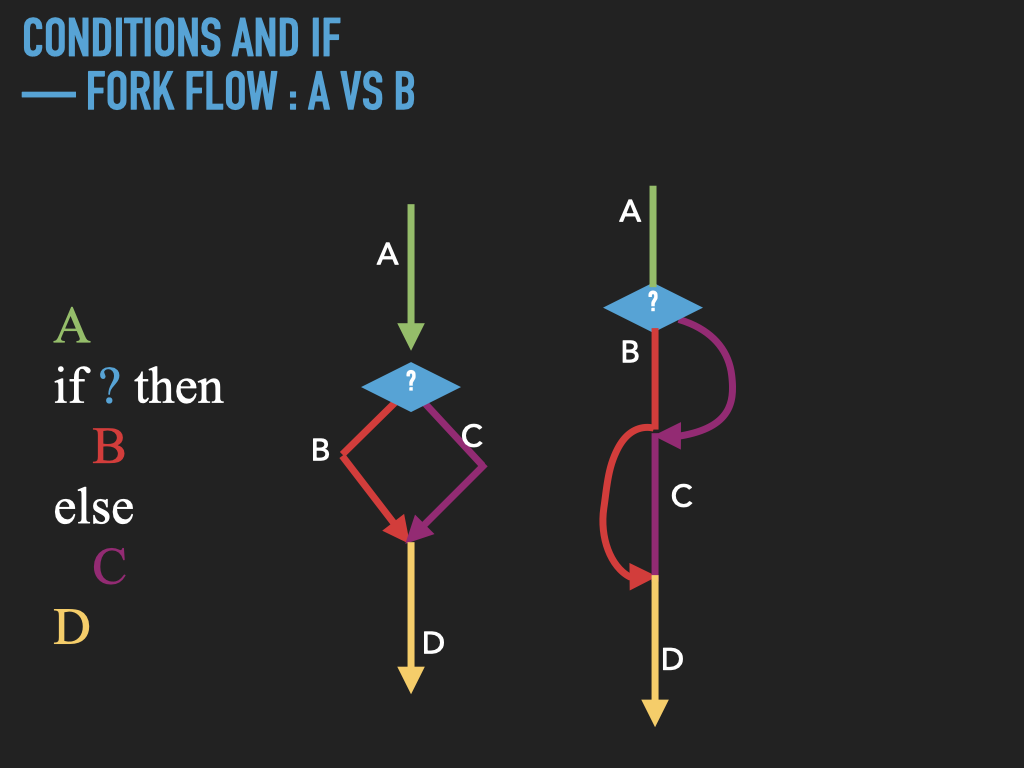
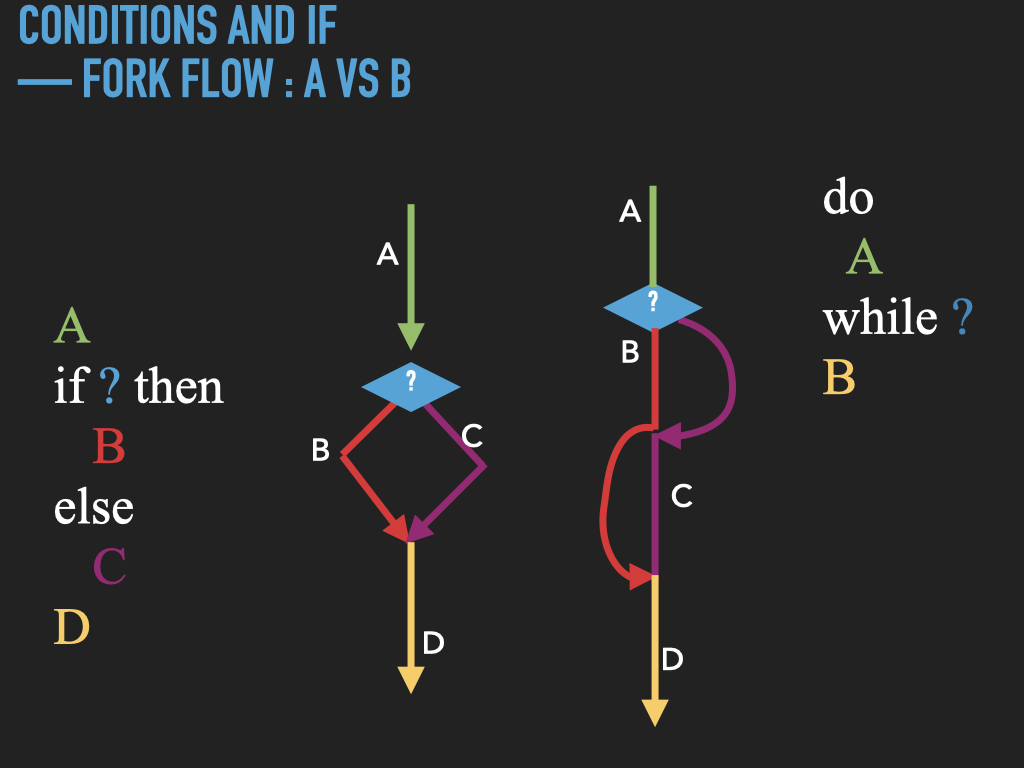
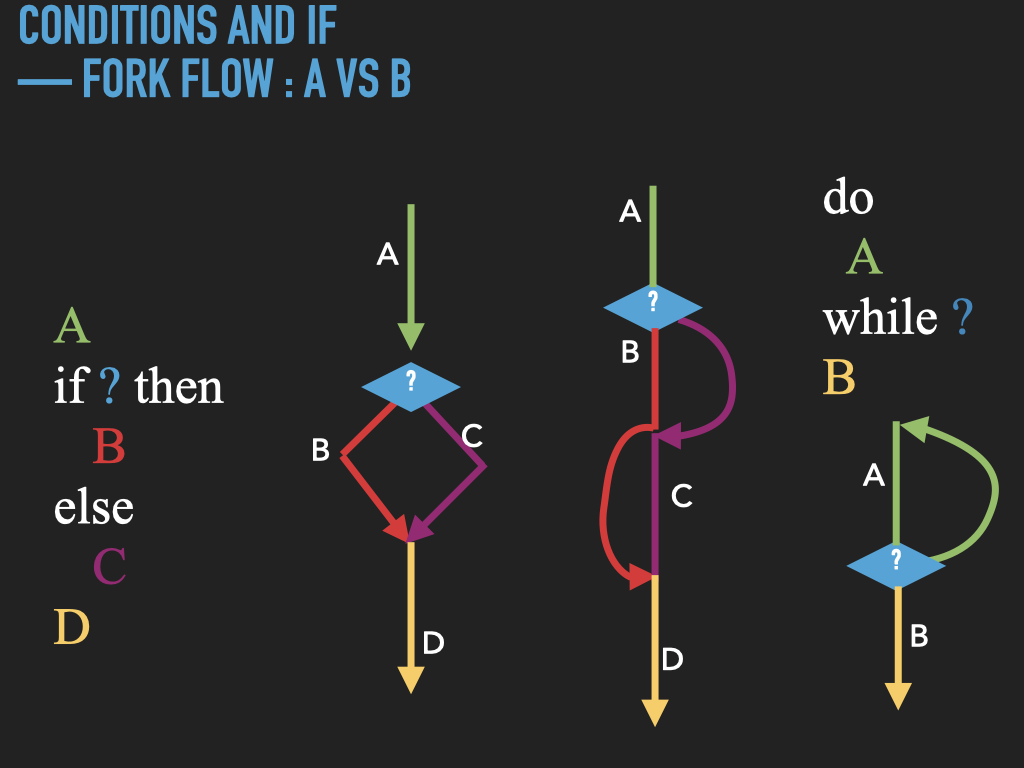
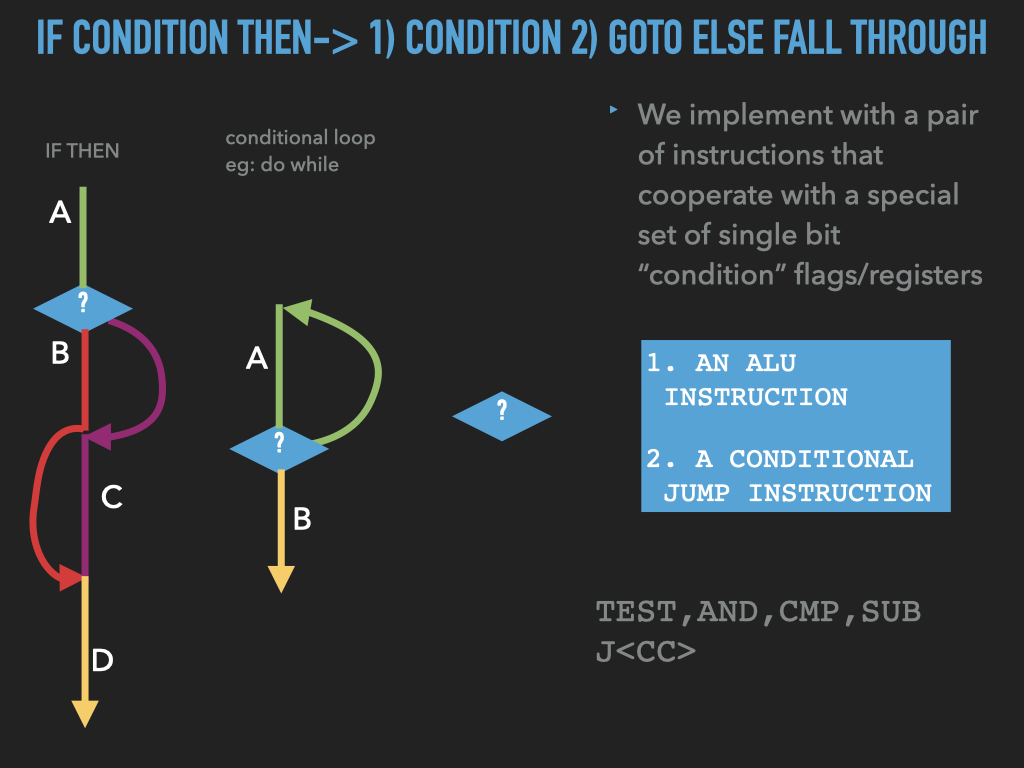
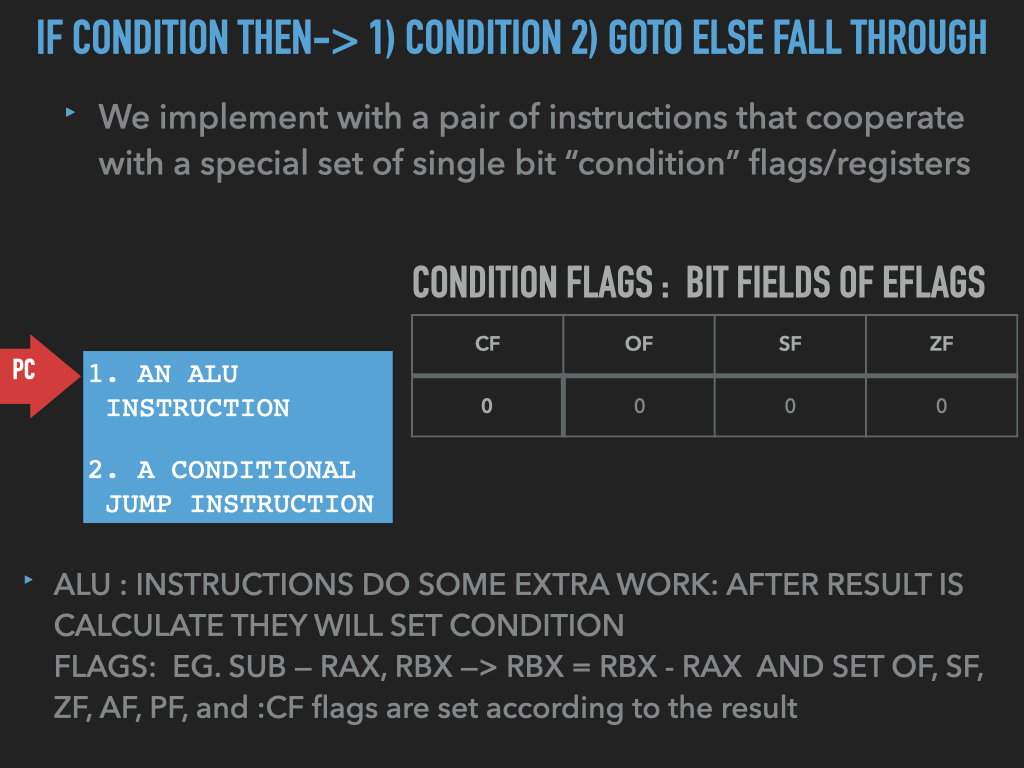
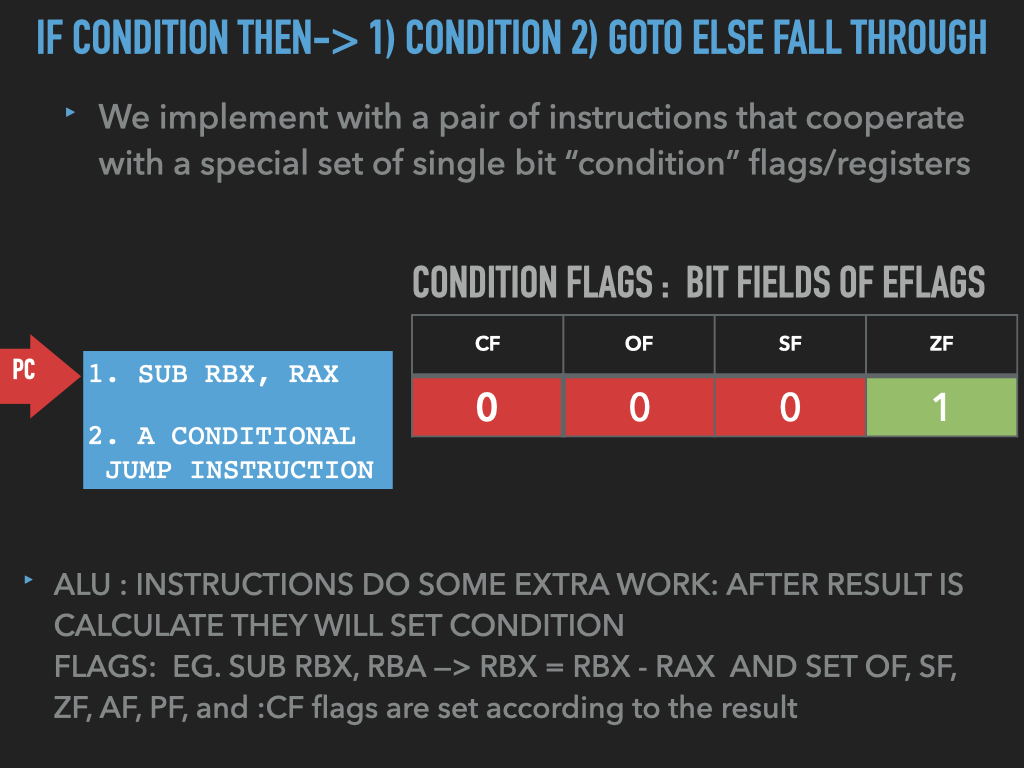
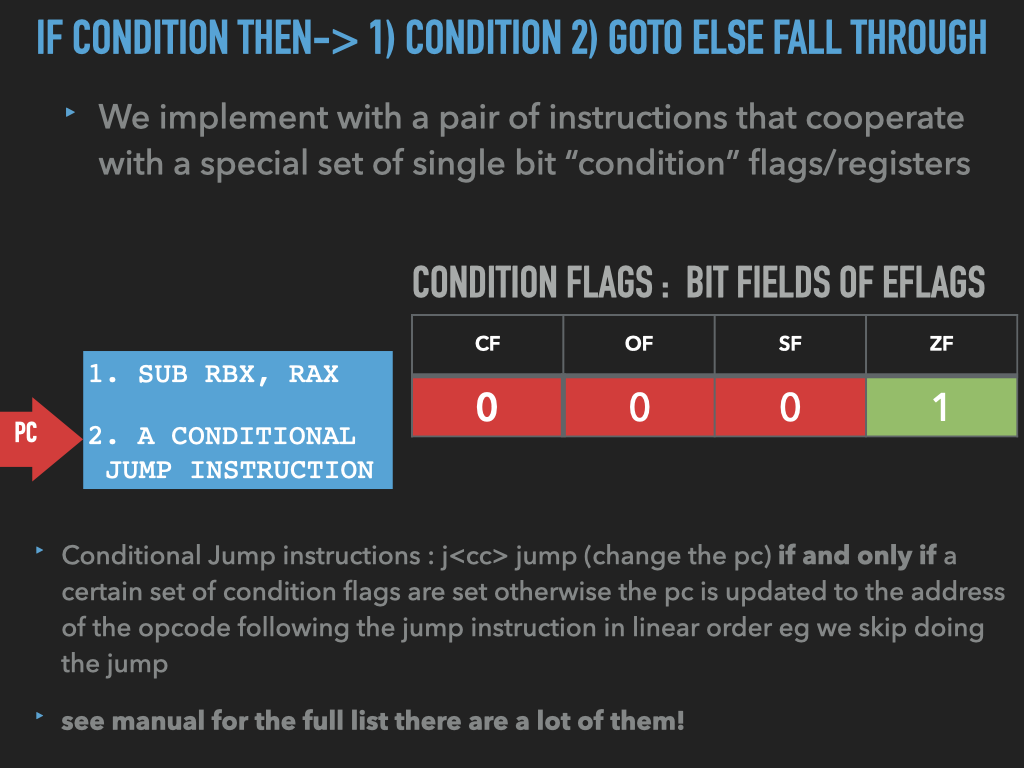
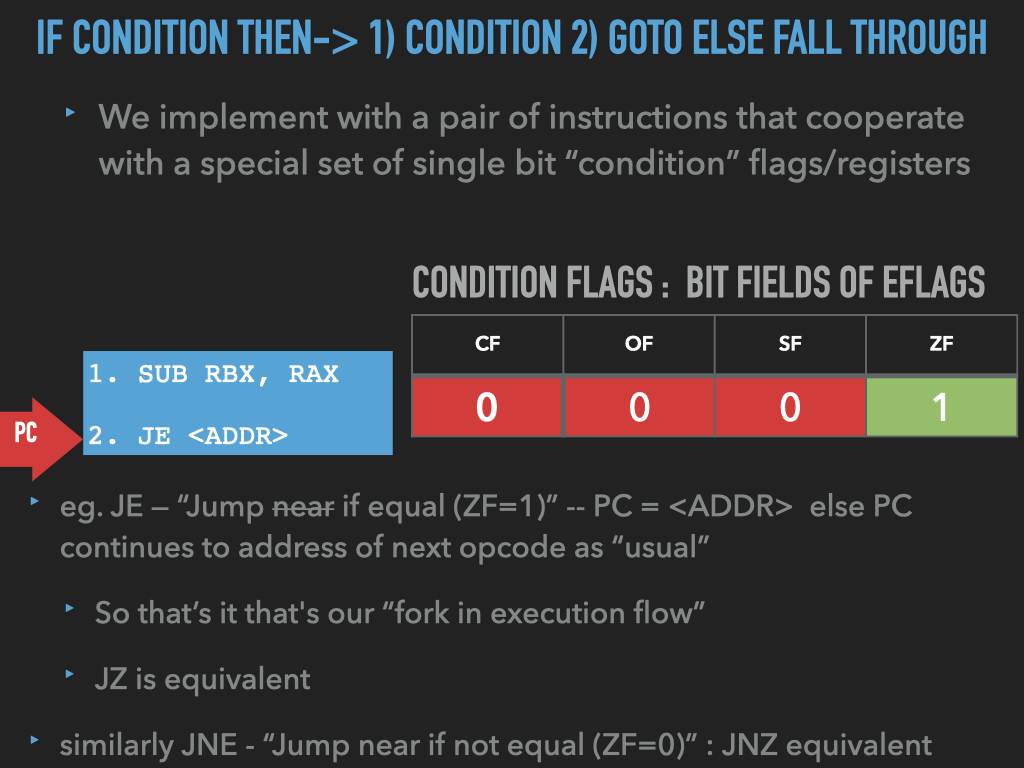
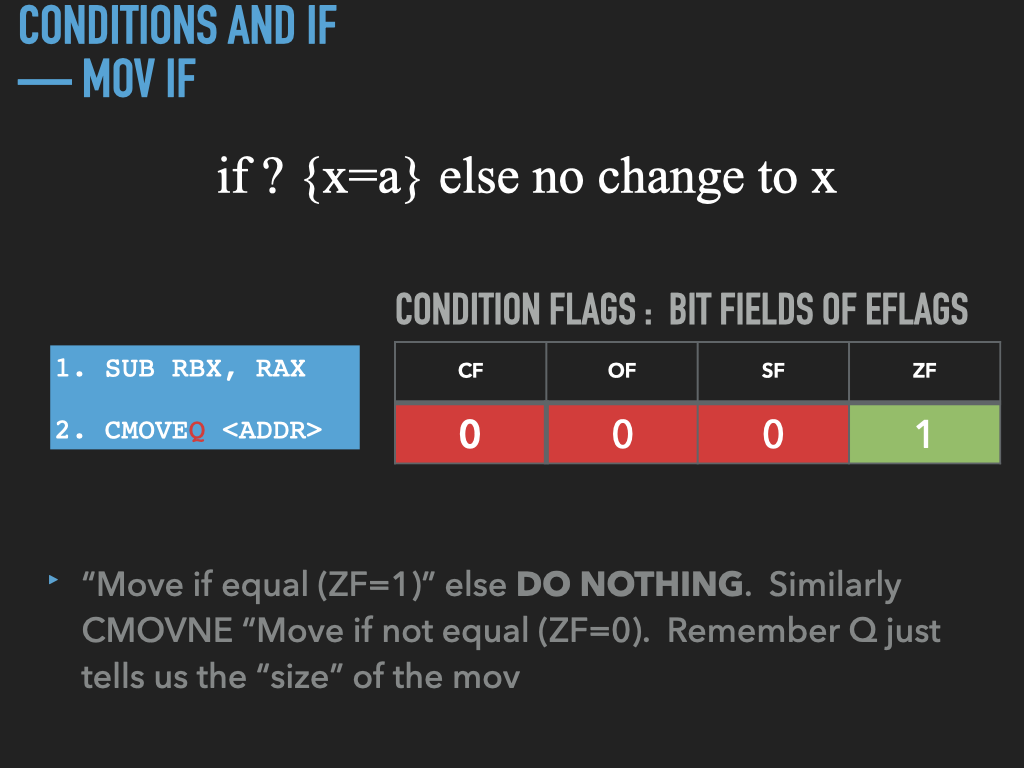
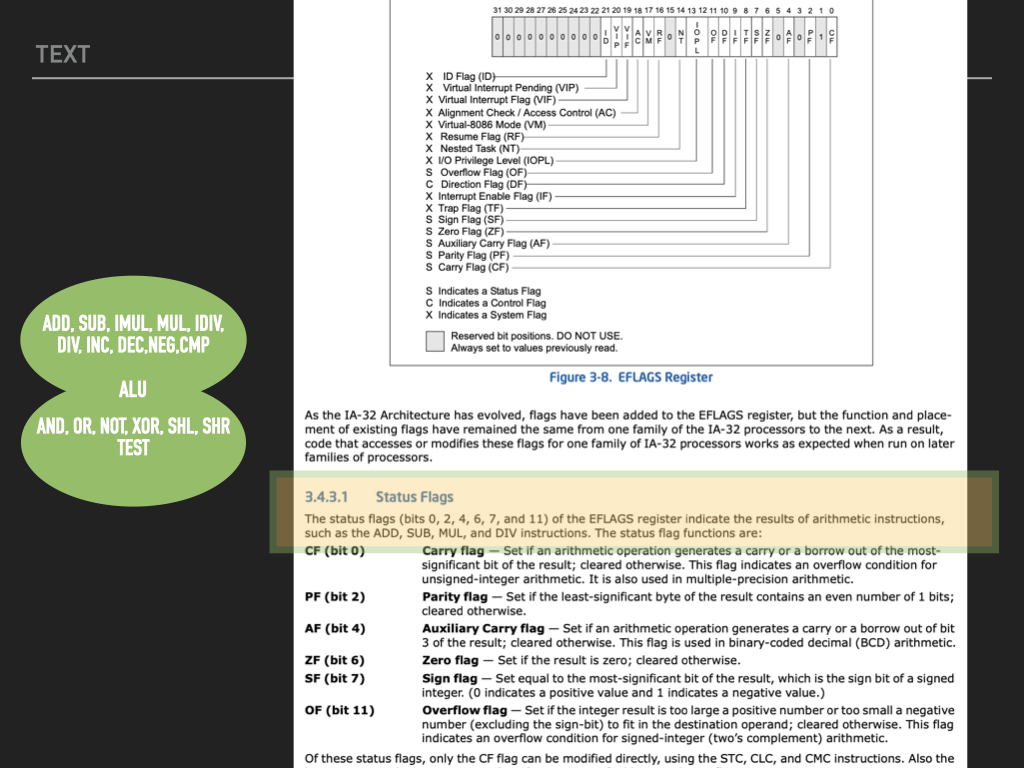
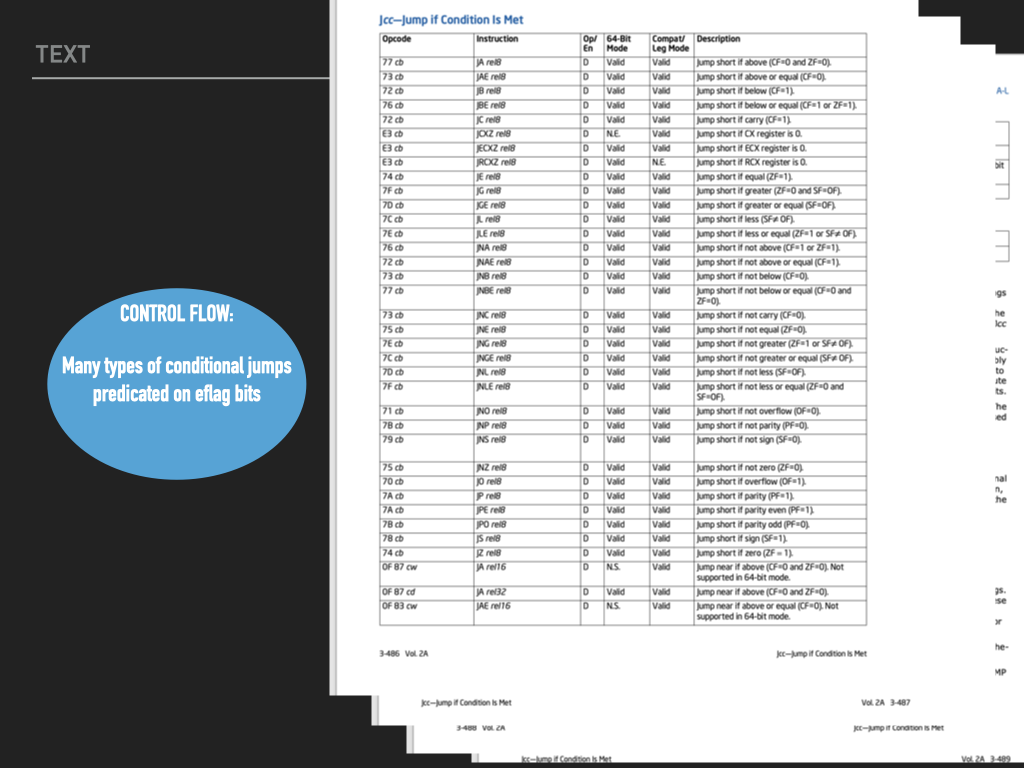
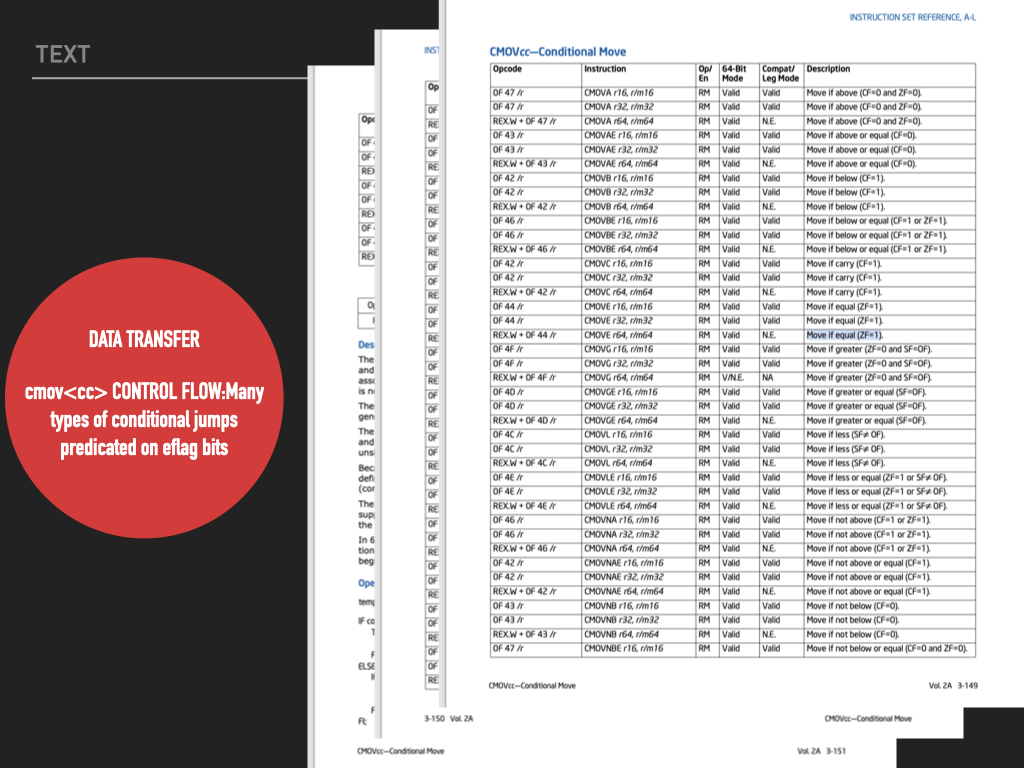
10.1. sumit.s
and usesum.s
#
10.1.1. Setup#
create a directory
mkdir sum; cd sum
create and write
sumit.s
andusesumit.s
see belowadd a
Makefile
to automate assembling and linkingwe are going run the commands by hand this time to highlight the details
add our
setup.gdb
to make working in gdb easiernormally you would want to track everything in git
10.1.2. Lets try and write a reusable routine#
lets assume that we have a symbol
XARRAY
that is the address of the datalets assume to use our routine you need to pass the length of the array
len in
rbx
use
rdi
for loop index (i
)
let put the result in
rax
Think about our objective in these terms
right?
Ok remember the tricky part is realizing that it is up to us to implement the idea of an array.
it is a data structure that we need to keep straight our head
CODE: asm - sumit.s
.intel_syntax noprefix
.section .text
# tell linker that sumIt symbol can be referenced in other files
.global sumIt
# code to sum data at XARRAY
# we assume rbx has length rbx -> len
# and that we will leave final sum in rax
sumIt: # label that marks where this code begins
xor rax, rax # rax -> sum : sum = 0
xor rdi, rdi # rdi -> i : i = 0
# code to sum data at XARRAY
# we assume rbx has length rbx -> len
# and that we will leave final sum in rax
loop_start:
cmp rbx, rdi # rbx - rdi
jz loop_done # if above is zero (they are equal) jump
add rax, QWORD PTR [XARRAY + rdi * 8] # add the i'th value to the sum
inc rdi # i=i+1
jmp loop_start # go back to the start of the loop
loop_done:
int3 # don't know where to go next
# give up and "trap" to the debugger
10.1.2.1. To assemble sumit.s
into sumit.o
#
10.1.3. So how might we use our “fragment”#
Lets create a program that defines a _start
routine and creates the memory locations that we can control.
Lets create usesum.s
Lets assume that
will set aside enough memory for an maximum of 1000 values in where we set the
XARRAY
symbolwe will allow the length actual length of data in
XARRAY
to be specified at a location marked byXARRAY_LEN
.we will store the result in a location marked by the symbol
sum
We will use our code by loading our data at XARRAY, updating XARRAY_LEN, executing the code and examining the result
The code should setup the memory we need
setup the registers as needed for
sumIt
run
sumIt
store the results at the location of
sum
CODE: asm - usesum.s
.intel_syntax noprefix
.section .data
# a place for us to store how much data is in the XARRAY
# initalized it to 0
XARRAY_LEN:
.quad 0x0
# reserve enough space for 1024 8 byte values
# third argument is alignment.... turns out cpu
# cpu is more efficient if things are located at address
# of a particular 'alignment (see intel manual)
.comm XARRAY, 8*1024, 8
.comm sum, 8, 8 # space to store final sum
.section .text
.global _start
_start:
mov rbx, QWORD PTR [XARRAY_LEN]
jmp sumIt
mov QWORD PTR [sum], rax
int3
10.1.3.1. To assemble usesum.s
into usesum.o
#
10.1.3.2. To link usesum.o
and sumit.o
into an executable usesum
#
10.1.4. Lets make some data!#
Lets create an ascii file with 10 numbers and then use a tool called ascii2binary
to convert it into 8 byte signed integers
10.1.4.1. Let’s make some “real” data#
Some Unix tricks of the trade
10.1.5. How to run usesum
and load data with gdb#
gdb -x setup.gdb usesum
b _start
run
# restore lets us load memory from a file
restore 10num.bin binary &XARRAY
# set the number of elements
set {long long} & XARRAY_LEN = 10
# get address of XARRAY
p & XARRAY
# now use this address to display the element we are currently summing
# eg lets assume the prior command indicated that the address of XARRAY is 0x402010
display {long long}(0x402010 + ($rdi * 8))
# display the XARRAY_LEN and sum so we can see if and when they change
display {{long long} & XARRAY_LEN, {long long} & sum}
# now we can single step our way through or continue till we hit an int3
You can do the same now with the 100randomnum.bin
file. Eg.restore 100randomnum.bin & XARRAY
and set {long long} & XARRAY_LEN = 100